在Linux系统中 编写利用消息缓冲通信方式,可实现消息的发送与接收,编写发送进程sndfile.c将要发送的消息从键盘输入,每输入一行就作为一条消息发送,用end结束消息,编写接收进程rcvfile.c从消息队里逐个接收信息并显示,用end结束消息。怎么写
时间: 2024-02-23 16:00:05 浏览: 133
好的,根据您的需求,我为您提供一份示例代码,其中包括了发送进程sndfile.c和接收进程rcvfile.c的代码。您可以根据需要进行修改和调整,以符合实际情况。
sndfile.c代码如下:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/msg.h>
#define MAX_SIZE 1024
struct msgbuf {
long mtype;
char mtext[MAX_SIZE];
};
int main()
{
key_t key;
int msgid;
struct msgbuf buf;
// 创建消息队列
key = ftok(".", 'a');
msgid = msgget(key, IPC_CREAT|0666);
if (msgid == -1) {
perror("msgget");
exit(EXIT_FAILURE);
}
// 从键盘输入消息并发送
printf("Please input the message:\n");
while (fgets(buf.mtext, MAX_SIZE, stdin) != NULL) {
// 将换行符替换为结束符
int len = strlen(buf.mtext);
if (buf.mtext[len-1] == '\n') {
buf.mtext[len-1] = '\0';
}
// 设置消息类型为1
buf.mtype = 1;
// 发送消息
if (msgsnd(msgid, (void*)&buf, MAX_SIZE, 0) == -1) {
perror("msgsnd");
exit(EXIT_FAILURE);
}
// 判断是否结束
if (strcmp(buf.mtext, "end") == 0) {
break;
}
// 清空消息缓冲区
memset(buf.mtext, 0, MAX_SIZE);
}
// 删除消息队列
if (msgctl(msgid, IPC_RMID, NULL) == -1) {
perror("msgctl");
exit(EXIT_FAILURE);
}
return 0;
}
```
rcvfile.c代码如下:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/msg.h>
#define MAX_SIZE 1024
struct msgbuf {
long mtype;
char mtext[MAX_SIZE];
};
int main()
{
key_t key;
int msgid;
struct msgbuf buf;
// 创建消息队列
key = ftok(".", 'a');
msgid = msgget(key, IPC_CREAT|0666);
if (msgid == -1) {
perror("msgget");
exit(EXIT_FAILURE);
}
// 接收消息并显示
while (1) {
// 接收消息
if (msgrcv(msgid, (void*)&buf, MAX_SIZE, 1, 0) == -1) {
perror("msgrcv");
exit(EXIT_FAILURE);
}
// 判断是否结束
if (strcmp(buf.mtext, "end") == 0) {
break;
}
// 显示消息
printf("Received message: %s\n", buf.mtext);
// 清空消息缓冲区
memset(buf.mtext, 0, MAX_SIZE);
}
// 删除消息队列
if (msgctl(msgid, IPC_RMID, NULL) == -1) {
perror("msgctl");
exit(EXIT_FAILURE);
}
return 0;
}
```
您可以将以上代码分别保存为sndfile.c和rcvfile.c文件,并在Linux系统中编译运行。其中,编译命令如下:
```
gcc -o sndfile sndfile.c
gcc -o rcvfile rcvfile.c
```
运行命令如下:
```
./sndfile
./rcvfile
```
请注意,以上代码仅供参考,具体实现可能需要根据实际情况进行修改和调整。同时,代码中未对输入内容进行安全性验证,可能存在安全风险,请您谨慎使用。
阅读全文
相关推荐
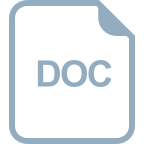
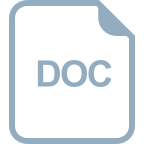
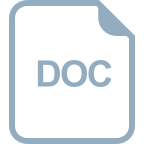


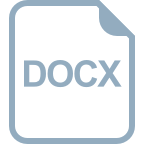
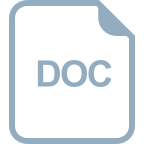
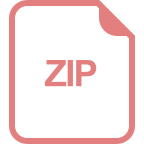
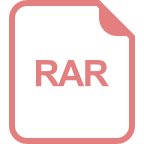








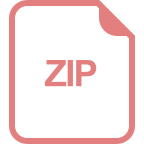
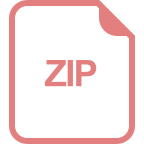