MATLAB读取32位tif格式灰度图,并转化为OpenCV支持的格式
时间: 2024-02-11 18:06:18 浏览: 28
MATLAB 通过 `imread` 函数可以读取 TIFF 格式的灰度图像,但是默认情况下,读取的 32 位灰度图像会被解释为浮点型图像,而不是整型图像。因此,在使用 OpenCV 处理这些图像之前,需要将其转换为整型图像。
下面是一个示例代码,演示了如何使用 MATLAB 读取 32 位 TIFF 格式的灰度图像,并将其转换为 OpenCV 支持的 8 位灰度图像格式:
```matlab
% 读取 32 位灰度图像
img = imread('test.tif');
% 将 32 位灰度图像转化为 8 位灰度图像
img = double(img);
img = (img / max(img(:))) * 255;
img = uint8(img);
% 将 MATLAB 数组转化为 OpenCV 格式的图像
cv_img = uint8(zeros(size(img, 1), size(img, 2), 3));
cv_img(:, :, 1) = img;
cv_img(:, :, 2) = img;
cv_img(:, :, 3) = img;
cv_img = cvtColor(cv_img, cv2.COLOR_BGR2GRAY);
% 显示结果
imshow(cv_img);
```
注意,上述代码中的转换方法仅适用于像素值在 0-65535 范围内的 32 位灰度图像。如果像素值超出了该范围,可能需要使用其他的转换方法。
相关问题
Python使用 Pillow库读取32位tif格式灰度图,并转化为OpenCV支持的格式
Pillow 库可以读取 32 位 TIFF 格式的图像,但是 OpenCV 不支持直接读取 32 位灰度图像。因此,在使用 OpenCV 处理 32 位灰度图像之前,需要将其转换为 8 位灰度图像。
下面是一个示例代码,演示了如何使用 Pillow 库读取 32 位 TIFF 格式的灰度图像,并将其转换为 OpenCV 支持的 8 位灰度图像格式:
```python
from PIL import Image
import numpy as np
import cv2
# 读取 32 位灰度图像
img = Image.open('test.tif')
img = img.convert('I') # 转化为 32 位整型灰度图像
arr = np.array(img) # 转化为 numpy 数组
# 将 32 位灰度图像转化为 8 位灰度图像
arr = arr.astype(np.float32) # 转化为浮点型数组
arr = (arr / arr.max()) * 255 # 线性拉伸到 0-255 范围内
arr = arr.astype(np.uint8) # 转化为无符号整型数组
# 将 numpy 数组转化为 OpenCV 格式的图像
cv_img = cv2.cvtColor(arr, cv2.COLOR_GRAY2BGR) # 将灰度图像转化为 BGR 格式
cv_img = cv2.cvtColor(cv_img, cv2.COLOR_BGR2GRAY) # 将 BGR 格式转化为灰度图像
# 显示结果
cv2.imshow('result', cv_img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
注意,上述代码中的转换方法仅适用于像素值在 0-65535 范围内的 32 位灰度图像。如果像素值超出了该范围,可能需要使用其他的转换方法。
Python和OpenCV将tif格式的灰度图转换为jpg
可以使用Python中的OpenCV库来将tif格式的灰度图转换为jpg。下面是一个示例代码:
```python
import cv2
# 加载tif格式图像
img = cv2.imread('image.tif', cv2.IMREAD_GRAYSCALE)
# 将图像转换为jpg格式并保存
cv2.imwrite('image.jpg', img, [int(cv2.IMWRITE_JPEG_QUALITY), 90])
```
在上面的代码中,`cv2.imread()`函数用于加载tif格式的灰度图像,`cv2.IMREAD_GRAYSCALE`参数指定了以灰度模式加载图像。然后,`cv2.imwrite()`函数将图像转换为jpg格式并保存到本地,其中第三个参数指定了图像压缩质量,默认值为95。
注意:在执行上述代码之前,需要先安装OpenCV库。可以使用pip命令来安装:`pip install opencv-python`。
相关推荐
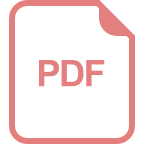
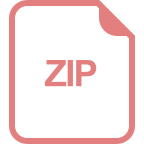












