生成一段关于优惠券使用的逻辑代码 使用vue
时间: 2024-03-16 10:46:07 浏览: 85
假设你正在使用 Vue.js 构建一个电商网站,下面是一个基于 Vue.js 的优惠券使用逻辑代码示例:
```vue
<template>
<div>
<input type="text" v-model="couponCode">
<button @click="handleCoupon">使用优惠券</button>
<div v-if="discountPrice">优惠金额:{{ discountPrice }} 元</div>
</div>
</template>
<script>
export default {
data() {
return {
couponCode: '',
couponInfo: null,
discountPrice: null,
};
},
methods: {
async handleCoupon() {
if (!this.couponCode) {
alert('请输入优惠码');
return;
}
try {
const res = await this.queryCouponInfo(this.couponCode);
if (!res) {
alert('优惠券不存在');
return;
}
if (res.expired) {
alert('优惠券已过期');
return;
}
if (this.totalPrice < res.minAmount) {
alert('订单金额不足');
return;
}
if (res.discountType === 'discount') {
if (res.discountAmount >= this.totalPrice) {
alert('优惠券抵扣金额大于订单金额,订单金额为 0 元');
this.discountPrice = 0;
} else {
this.discountPrice = res.discountAmount;
alert(`优惠券抵扣 ${this.discountPrice} 元`);
}
} else if (res.discountType === 'reduce') {
this.discountPrice = res.discountAmount;
alert(`优惠券抵扣 ${this.discountPrice} 元`);
} else {
alert('优惠券类型错误');
return;
}
} catch (error) {
alert('查询优惠券信息失败');
console.error(error);
}
},
async queryCouponInfo(couponCode) {
// 发送请求查询优惠券信息
// 返回优惠券信息
},
},
};
</script>
```
在上面的代码中,我们首先使用 v-model 指令将输入框的值绑定到 couponCode 变量上,然后在点击按钮时调用 handleCoupon 方法来处理优惠券的使用。
在 handleCoupon 方法中,我们首先判断是否输入了优惠码,然后调用 queryCouponInfo 方法查询优惠券信息。如果优惠券不存在或者已经过期或者订单金额不足,则给出相应的错误提示。
如果优惠券类型是折扣类型,则判断抵扣金额是否大于订单金额,如果是则订单金额为 0 元,否则设置 discountPrice 变量为抵扣金额。如果优惠券类型是满减类型,则直接设置 discountPrice 变量为抵扣金额。
最后,在页面上显示 discountPrice 变量的值,如果值为 null 则不显示该元素。
以上是一个基于 Vue.js 的优惠券使用逻辑代码示例,具体实现方式可能会因业务需求不同而有所差异。
阅读全文
相关推荐
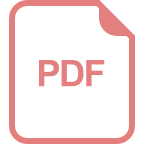
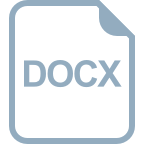
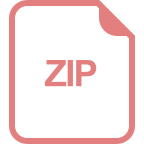
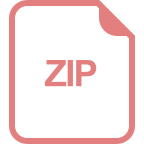
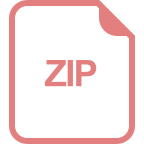
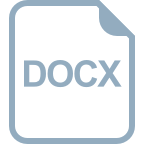
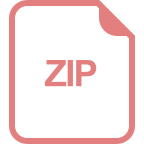
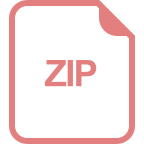