十大优秀青年评比基于C语言的代码
时间: 2024-05-01 11:22:53 浏览: 4
很抱歉,我不太明白你的意思。你是想要我给出一些基于C语言的优秀代码示例吗?如果是的话,以下是一些可以参考的代码片段:
1. 实现二分查找算法:
```
int binary_search(int arr[], int n, int key) {
int low = 0, high = n - 1;
while (low <= high) {
int mid = (low + high) / 2;
if (arr[mid] == key)
return mid;
else if (arr[mid] < key)
low = mid + 1;
else
high = mid - 1;
}
return -1;
}
```
2. 实现快速排序算法:
```
void quick_sort(int arr[], int left, int right) {
if (left >= right)
return;
int i = left, j = right, pivot = arr[left];
while (i < j) {
while (i < j && arr[j] >= pivot)
j--;
if (i < j)
arr[i++] = arr[j];
while (i < j && arr[i] < pivot)
i++;
if (i < j)
arr[j--] = arr[i];
}
arr[i] = pivot;
quick_sort(arr, left, i - 1);
quick_sort(arr, i + 1, right);
}
```
3. 实现链表数据结构:
```
struct ListNode {
int val;
struct ListNode *next;
};
struct ListNode* create_list(int arr[], int n) {
struct ListNode *head = NULL, *tail = NULL;
for (int i = 0; i < n; i++) {
struct ListNode *node = (struct ListNode*)malloc(sizeof(struct ListNode));
node->val = arr[i];
node->next = NULL;
if (!head) {
head = tail = node;
} else {
tail->next = node;
tail = node;
}
}
return head;
}
```
4. 实现栈数据结构:
```
#define MAX_SIZE 100
struct Stack {
int data[MAX_SIZE];
int top;
};
void init_stack(struct Stack* s) {
s->top = -1;
}
int is_empty(struct Stack* s) {
return s->top == -1;
}
int is_full(struct Stack* s) {
return s->top == MAX_SIZE - 1;
}
void push(struct Stack* s, int x) {
if (is_full(s))
return;
s->data[++s->top] = x;
}
int pop(struct Stack* s) {
if (is_empty(s))
return -1;
return s->data[s->top--];
}
```
5. 实现队列数据结构:
```
#define MAX_SIZE 100
struct Queue {
int data[MAX_SIZE];
int front, rear;
};
void init_queue(struct Queue* q) {
q->front = q->rear = -1;
}
int is_empty(struct Queue* q) {
return q->front == -1;
}
int is_full(struct Queue* q) {
return (q->rear + 1) % MAX_SIZE == q->front;
}
void enqueue(struct Queue* q, int x) {
if (is_full(q))
return;
if (is_empty(q))
q->front = q->rear = 0;
else
q->rear = (q->rear + 1) % MAX_SIZE;
q->data[q->rear] = x;
}
int dequeue(struct Queue* q) {
if (is_empty(q))
return -1;
int x = q->data[q->front];
if (q->front == q->rear)
q->front = q->rear = -1;
else
q->front = (q->front + 1) % MAX_SIZE;
return x;
}
```
以上是一些基于C语言的代码示例,希望能对你有所帮助。如果你有其他问题,请随时问我。
相关推荐
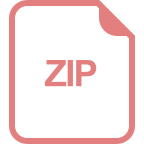
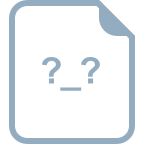
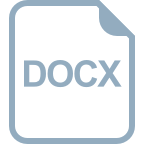














