爬取网易云音乐 Top105,存至 mysql 数据库中
时间: 2024-04-28 09:23:19 浏览: 8
好的,我可以为您提供一个大致的思路:
1. 使用 Python 的 requests 和 BeautifulSoup 库爬取网易云音乐 Top105 的页面,获取每首歌曲的排名、歌曲名、歌手和歌曲 ID 等信息。
2. 使用 Python 的 mysql-connector 库连接到 MySQL 数据库,创建一个表来存储这些信息。
3. 遍历每首歌曲的信息,将其插入到数据库表中。可以使用 SQL 语句的 INSERT INTO 命令来实现。
下面是一个简单的示例代码,仅供参考:
```python
import requests
from bs4 import BeautifulSoup
import mysql.connector
# 爬取 Top105 页面
url = 'https://music.163.com/discover/toplist?id=3778678'
r = requests.get(url)
soup = BeautifulSoup(r.text, 'html.parser')
# 连接到 MySQL 数据库
cnx = mysql.connector.connect(user='yourusername', password='yourpassword',
host='127.0.0.1', database='yourdatabase')
cursor = cnx.cursor()
# 创建一个表来存储歌曲信息
create_table = """
CREATE TABLE IF NOT EXISTS Top105 (
id INT(11) NOT NULL AUTO_INCREMENT,
rank INT(11),
title VARCHAR(255),
artist VARCHAR(255),
song_id VARCHAR(255),
PRIMARY KEY (id)
)
"""
cursor.execute(create_table)
# 遍历每首歌曲的信息,将其插入到数据库表中
songs = soup.select('ul.f-hide li')
for song in songs:
rank = song.select_one('span').text.strip()
title = song.select_one('a').text.strip()
artist = song.select_one('span:nth-of-type(2)').text.strip()
song_id = song.select_one('a')['href'].split('=')[-1]
insert_song = f"INSERT INTO Top105 (rank, title, artist, song_id) VALUES ({rank}, '{title}', '{artist}', '{song_id}')"
cursor.execute(insert_song)
# 提交更改和关闭连接
cnx.commit()
cursor.close()
cnx.close()
```
需要注意的是,此代码仅供参考,具体实现可能需要根据您的需求进行修改。同时,由于网易云音乐的反爬虫机制较为严格,因此在实际使用时需要注意不要过于频繁地访问页面,以免被封禁 IP。
相关推荐
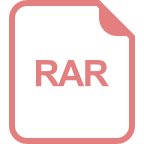
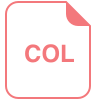
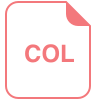












