在c语言中,将若干字符串按照宇母顺序 (由小到大)输出,用指针数组的方法来写出
时间: 2024-12-23 14:20:02 浏览: 8
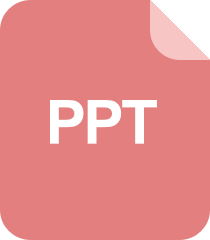
指针数组的应用-C语言指针课程资源
在C语言中,如果你想按照字母顺序(从小到大)输出若干个字符串,可以使用字符数组存储每个字符串,并通过指针数组来访问它们。首先,你需要定义一个字符指针数组来存放字符串,然后创建一个结构体来包含字符串及其对应的索引。这里假设我们有一个字符串数组`strs[]`和它们的长度数组`len[]`。
```c
#include <stdio.h>
#include <string.h>
// 定义结构体来存储字符串及其索引
typedef struct {
char* str;
int index; // 或者使用size_t代替int
} StringInfo;
void sortStrings(char** strs, int len[], int num) {
// 比较函数,用于qsort排序
int compare(const void *a, const void *b) {
StringInfo* sa = (StringInfo*) a;
StringInfo* sb = (StringInfo*) b;
return strcmp(sa->str, sb->str);
}
// 使用快速排序算法对字符串信息进行排序
qsort(strs, num, sizeof(StringInfo), compare);
// 输出排序后的字符串
for (int i = 0; i < num; ++i) {
printf("%d: %s\n", i, strs[i]->str); // 如果需要保持原索引,替换为printf("%d: %s\n", strs[i].index, strs[i]->str);
}
}
int main() {
char* strs[] = {"hello", "world", "apple", "banana"};
int len[] = {5, 5, 5, 6};
int num = sizeof(strs) / sizeof(strs[0]);
sortStrings(strs, len, num);
return 0;
}
```
阅读全文
相关推荐
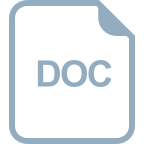
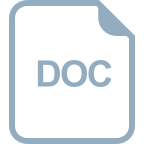
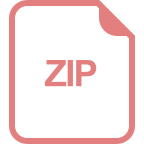
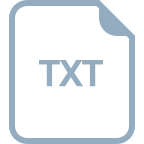
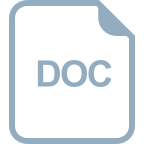
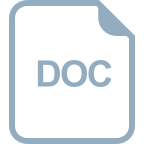
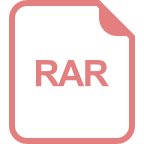
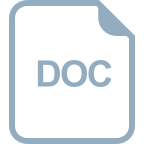
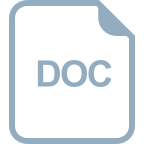
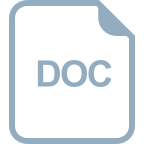
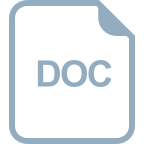
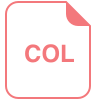
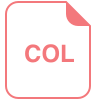
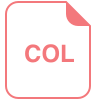
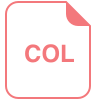
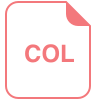
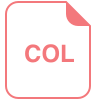
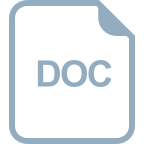