小程序使用ocr的代码
时间: 2024-06-09 12:11:24 浏览: 174
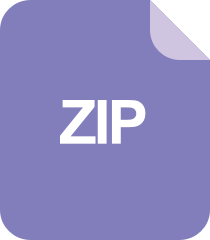
OCR识别小程序

以下是使用百度AI开放平台的OCR API实现小程序中OCR功能的代码示例:
```javascript
// 在小程序中使用百度OCR API进行OCR识别
const appKey = 'your_app_key'; // 应用的API Key
const secretKey = 'your_secret_key'; // 应用的Secret Key
const ocrUrl = 'https://aip.baidubce.com/rest/2.0/ocr/v1/general_basic'; // OCR API的URL
// 封装发送请求的方法
function request(url, method, header = {}, data = {}) {
return new Promise((resolve, reject) => {
wx.request({
url: url,
method: method,
header: header,
data: data,
success: res => {
resolve(res.data);
},
fail: error => {
reject(error);
}
})
})
}
// 封装获取OCR Token的方法
function getOcrToken() {
const url = `https://aip.baidubce.com/oauth/2.0/token?grant_type=client_credentials&client_id=${appKey}&client_secret=${secretKey}`;
return request(url, 'GET');
}
// 封装使用OCR API进行识别的方法
async function ocrImage(imageUrl) {
const tokenData = await getOcrToken();
const token = tokenData.access_token;
const header = {
'Content-Type': 'application/x-www-form-urlencoded',
};
const data = {
image: imageUrl,
access_token: token,
};
const result = await request(ocrUrl, 'POST', header, data);
return result.words_result;
}
// 在小程序中调用OCR API进行识别
wx.chooseImage({
count: 1, // 选择图片的数量
success: async function (res) {
const tempFilePath = res.tempFilePaths[0];
const imageData = await wx.getFileSystemManager().readFile({
filePath: tempFilePath,
encoding: 'base64'
});
const ocrResult = await ocrImage(imageData.data);
console.log(ocrResult);
}
})
```
注意:上述代码中需要替换自己应用的API Key和Secret Key。同时,代码中使用了`wx.chooseImage`方法选择图片,需要在小程序中调用该方法并选择一张图片进行OCR识别。
阅读全文
相关推荐
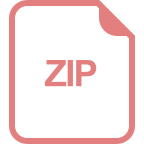
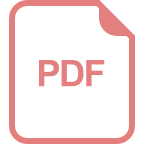















