plaese use C++ language You are given an unsorted permutation p1,p2,…,pn. To sort the permutation, you choose a constant k(k≥1) and do some operations on the permutation. In one operation, you can choose two integers i, j (1≤j<i≤n) such that i−j=k, then swap pi and pj.
时间: 2023-06-17 22:08:46 浏览: 54
Sure, here's a possible implementation in C++:
```
#include <iostream>
#include <vector>
using namespace std;
void sort_permutation(vector<int>& p) {
int n = p.size();
for (int k = 1; k <= n; k++) {
for (int i = k+1; i <= n; i++) {
if ((i-k) % k == 0) {
int j = i-k;
if (p[i-1] < p[j-1]) {
swap(p[i-1], p[j-1]);
}
}
}
}
}
int main() {
vector<int> p = {4, 2, 3, 1, 5};
sort_permutation(p);
for (int x : p) {
cout << x << " ";
}
cout << endl;
return 0;
}
```
The function `sort_permutation` takes a vector `p` representing an unsorted permutation, and sorts it by performing swaps between elements that are k positions apart, for all values of k from 1 to n. The main function shows an example usage with the permutation {4, 2, 3, 1, 5}. The output should be {1, 2, 3, 4, 5}.
相关推荐
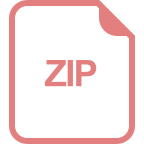
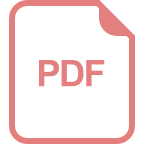
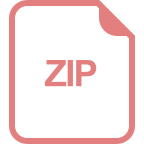
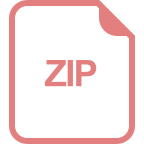
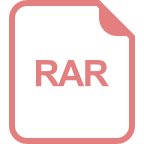
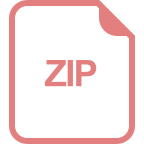
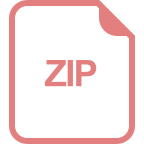
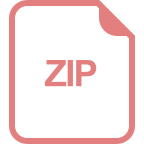
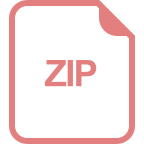