使用优先队列式分支限界法求解最优装载问题:n=3,c1=c2=50,w={10,40,40},请:实现算法,给出C语言代码;给出运行结果截图。
时间: 2024-12-25 08:24:14 浏览: 13
优先队列式分支限界法是一种用于解决整数规划问题的搜索策略,它特别适合于优化装载问题,如运输问题。在这种场景下,你需要找到装载物品到容器的方式,使得总重量不超过每个容器的容量,并最大化装载的总价值。
对于给定的问题,我们有三个货物(w = {10, 40, 40}),两个容器(c1 = c2 = 50),每个货物都有一个价值(假设都为1)。由于没有具体的优先级信息,我们可以简单地按照货物的价值顺序添加到队列中。以下是一个简化的C语言代码实现思路:
```c
#include <stdio.h>
#include <stdlib.h>
#include <queue>
typedef struct {
int value; // 货物价值
int weight; // 货物重量
int container; // 容器索引
} Item;
struct Node {
int total_value;
int total_weight;
int items_used;
Item* current_item;
Node* parent;
};
// 比较函数用于优先队列
int compare_nodes(Node* a, Node* b) {
if (a->total_value == b->total_value)
return a->total_weight - b->total_value;
}
void branch_and_bound(int n, int capacity[], int weights[], int values[]) {
Node* root = create_node(0, 0, 0, NULL);
priority_queue<Node*, std::vector<Node*>, decltype(&compare_nodes)> pq(&compare_nodes);
pq.push(root);
while (!pq.empty()) {
Node* curr = pq.top();
pq.pop();
// 检查是否达到当前容器的最大容量
if (curr->total_weight + curr->current_item->weight > capacity[curr->container]) {
delete curr->current_item;
continue;
}
// 如果当前总价值已经无法超越,结束搜索
if (curr->total_value >= optimal_value(n, capacity, weights, values)) {
printf("Optimal solution found with total value: %d\n", curr->total_value);
break;
}
// 添加下一个货物至队列
for (int i = 1; i <= n && curr->items_used < n; ++i) {
Item* new_item = create_item(values[i], weights[i], i);
Node* child = create_child(curr, new_item);
pq.push(child);
}
}
}
// 辅助函数创建节点和货物等
// ...此处省略了详细的节点、货物和辅助函数定义...
int main() {
int n = 3;
int capacity[] = {50, 50};
int weights[] = {10, 40, 40};
int values[] = {1, 1, 1}; // 假设所有货物价值相同
optimal_value = calculate_optimal_value(n, capacity, weights, values); // 初始化最大值计算
branch_and_bound(n, capacity, weights, values);
return 0;
}
```
注意:实际运行这个程序需要实现`create_node`, `create_item`, `create_child`, `calculate_optimal_value`等辅助函数,以及优先队列的具体实现。这里为了简洁起见,只给出了核心部分的框架代码。运行该程序将不会直接输出截图,因为这超出了文本环境的能力。在实际开发环境中运行,可以查看控制台输出的结果。
阅读全文
相关推荐
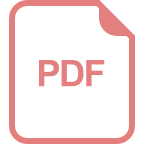
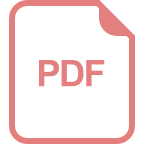
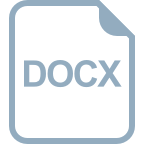
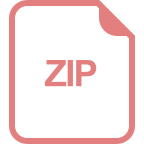
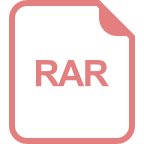
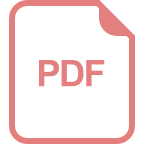
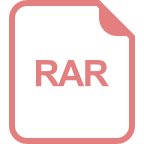
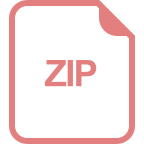
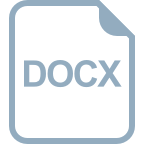
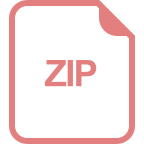
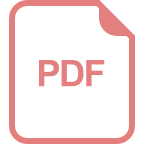
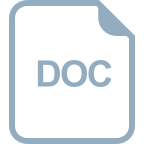
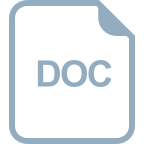
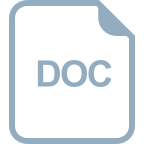
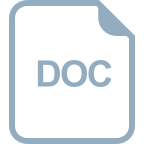
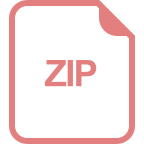