winform 串口接收到数据放置到List,对数据包进行解析,并用SCOTTPLOT绘制实时曲线,2ms刷新一次
时间: 2024-05-15 18:19:55 浏览: 173
以下是一个简单的示例代码,用于在WinForm中接收通过串口发送的数据,并将其放入List中进行解析和绘制实时曲线。该示例使用了ScottPlot库进行图形绘制和更新,每2ms刷新一次。
```csharp
using System;
using System.Collections.Generic;
using System.IO.Ports;
using System.Windows.Forms;
using ScottPlot;
namespace WinForm_Serialport_Data_Plot
{
public partial class Form1 : Form
{
private SerialPort serialPort;
private List<double> dataList = new List<double>();
private Plot plt;
public Form1()
{
InitializeComponent();
// 初始化串口
serialPort = new SerialPort("COM1", 9600, Parity.None, 8, StopBits.One);
serialPort.DataReceived += new SerialDataReceivedEventHandler(DataReceivedHandler);
serialPort.Open();
// 初始化图形
plt = new Plot(800, 400);
plt.PlotSignal(dataList.ToArray());
plt.YLabel("Data");
plt.XLabel("Time");
plt.Title("Real-time Data Plot");
plt.AxisAuto();
plt.Axis(-100, 0, -10, 10);
plt.Ticks(dateTimeX: true);
plt.MouseWheelZoomEnabled = false;
plt.RightClickMenuEnabled = false;
// 将图形添加到窗口中
this.Controls.Add(plt.GetBitmap());
}
private void DataReceivedHandler(object sender, SerialDataReceivedEventArgs e)
{
// 读取串口数据并将其添加到列表中
string data = serialPort.ReadLine();
double val = double.Parse(data);
dataList.Add(val);
}
private void timer1_Tick(object sender, EventArgs e)
{
// 更新图形
plt.Clear();
plt.PlotSignal(dataList.ToArray());
plt.AxisAuto();
plt.Axis(-100, 0, -10, 10);
plt.Ticks(dateTimeX: true);
plt.MouseWheelZoomEnabled = false;
plt.RightClickMenuEnabled = false;
// 更新图像到窗口中
plt.GetBitmap();
}
}
}
```
在窗口中,我们需要添加一个Timer组件,用于定时刷新图形。我们可以将其Interval属性设置为2,以便每2ms刷新一次图形。
在窗口中还需要添加一个Button组件,我们可以使用该按钮来启动定时器并开始显示实时数据。在点击该按钮时,我们可以将Timer组件的Enabled属性设置为true,以便开始定时器。
```csharp
private void button1_Click(object sender, EventArgs e)
{
timer1.Enabled = true;
}
```
当不再需要显示实时数据时,我们可以使用Stop按钮停止定时器并停止显示实时数据。在点击该按钮时,我们可以将Timer组件的Enabled属性设置为false,以便停止定时器。
```csharp
private void button2_Click(object sender, EventArgs e)
{
timer1.Enabled = false;
}
```
阅读全文
相关推荐
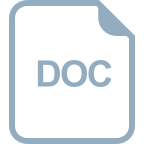
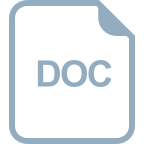
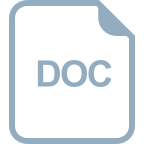


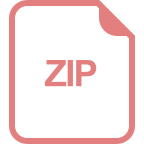
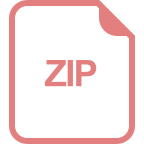
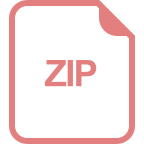
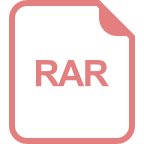
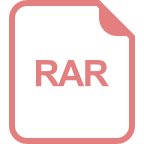
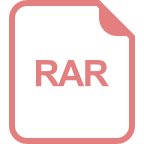
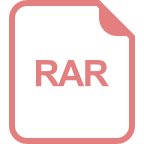
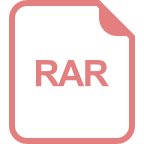
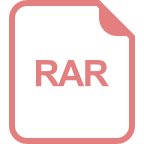
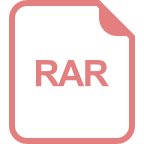
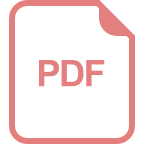
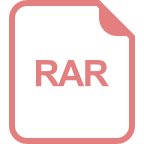