开发一个模拟HBase Shell命令行终端的应用程序实现其DDL分组和DML分组的常用操作命令。
时间: 2023-11-27 11:51:28 浏览: 70
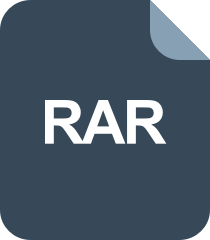
模拟的shell程序
好的,以下是一个简单的模拟HBase Shell命令行终端的应用程序,可以实现DDL分组和DML分组的常用操作命令:
```python
class HBaseShell:
def __init__(self):
self.tables = {} # 存储所有表的数据
self.current_table = None # 当前操作的表名
def create_table(self, table_name, column_families):
if table_name in self.tables:
print(f"Table '{table_name}' already exists.")
return
self.tables[table_name] = {}
for cf in column_families:
self.tables[table_name][cf] = {}
def list_tables(self):
print("TABLE")
print("------------------------------")
for table_name in self.tables.keys():
print(table_name)
def describe_table(self, table_name):
if table_name not in self.tables:
print(f"Table '{table_name}' does not exist.")
return
print(f"DESCRIPTION OF '{table_name}'")
print("------------------------------")
for cf in self.tables[table_name].keys():
print(cf)
def put(self, row_key, column_family, column, value):
if not self.current_table:
print("No table selected.")
return
if column_family not in self.tables[self.current_table]:
print(f"Column family '{column_family}' does not exist in table '{self.current_table}'.")
return
self.tables[self.current_table][column_family][f"{column}:{row_key}"] = value
def get(self, row_key):
if not self.current_table:
print("No table selected.")
return
for cf, columns in self.tables[self.current_table].items():
for column, value in columns.items():
if row_key in column:
print(f"{cf}:{column.split(':')[0]} {value}")
def delete(self, row_key, column_family=None, column=None):
if not self.current_table:
print("No table selected.")
return
if column_family and column:
del self.tables[self.current_table][column_family][f"{column}:{row_key}"]
elif column_family:
del self.tables[self.current_table][column_family]
else:
for cf in self.tables[self.current_table].keys():
for column in list(self.tables[self.current_table][cf].keys()):
if row_key in column:
del self.tables[self.current_table][cf][column]
def select_table(self, table_name):
if table_name not in self.tables:
print(f"Table '{table_name}' does not exist.")
return
self.current_table = table_name
def exit_shell(self):
self.current_table = None
print("Goodbye!")
def help(self):
print("""Available commands:
CREATE TABLE <table_name> <column_family1> <column_family2> ...
LIST TABLES
DESCRIBE <table_name>
PUT <row_key> <column_family> <column> <value>
GET <row_key>
DELETE <row_key> [<column_family> [<column>]]
SELECT <table_name>
EXIT
HELP""")
if __name__ == "__main__":
shell = HBaseShell()
while True:
command = input("hbase> ")
tokens = command.split()
if not tokens:
continue
if tokens[0].lower() == "create" and len(tokens) >= 4 and tokens[1].lower() == "table":
shell.create_table(tokens[2], tokens[3:])
elif tokens[0].lower() == "list" and tokens[1].lower() == "tables":
shell.list_tables()
elif tokens[0].lower() == "describe" and len(tokens) == 2:
shell.describe_table(tokens[1])
elif tokens[0].lower() == "put" and len(tokens) == 5:
shell.put(tokens[1], tokens[2], tokens[3], tokens[4])
elif tokens[0].lower() == "get" and len(tokens) == 2:
shell.get(tokens[1])
elif tokens[0].lower() == "delete" and len(tokens) >= 2:
if len(tokens) == 4:
shell.delete(tokens[1], tokens[2], tokens[3])
elif len(tokens) == 3:
shell.delete(tokens[1], tokens[2])
else:
shell.delete(tokens[1])
elif tokens[0].lower() == "select" and len(tokens) == 2:
shell.select_table(tokens[1])
elif tokens[0].lower() == "exit":
shell.exit_shell()
break
elif tokens[0].lower() == "help":
shell.help()
else:
print("Invalid command. Type 'help' for available commands.")
```
这个程序支持以下命令:
- `CREATE TABLE <table_name> <column_family1> <column_family2> ...`:创建一个新的表,包含指定的列族。
- `LIST TABLES`:列出所有已创建的表。
- `DESCRIBE <table_name>`:显示指定表的列族。
- `PUT <row_key> <column_family> <column> <value>`:将一个值存储到表中的指定行、列族和列。
- `GET <row_key>`:检索指定行的所有列族和列。
- `DELETE <row_key> [<column_family> [<column>]]`:删除指定行、列族和列的值。
- `SELECT <table_name>`:选择要操作的表。
- `EXIT`:退出程序。
- `HELP`:列出所有可用的命令。
注意:这个程序只是一个简单的演示,没有实现HBase的所有功能。在实际使用中,可能需要根据具体需求进行扩展。
阅读全文
相关推荐
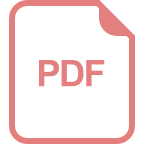
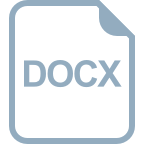
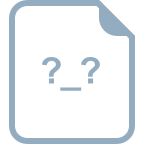
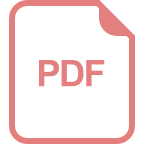
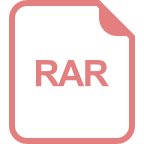
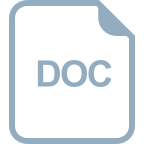
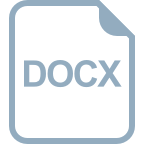
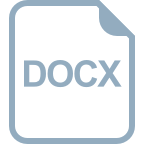
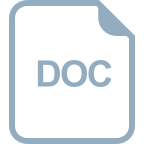
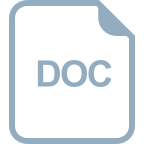
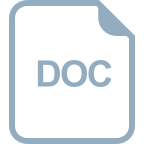
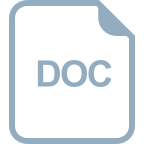
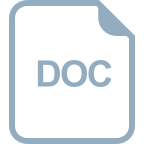
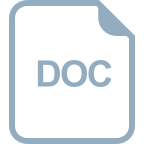
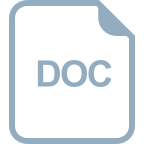
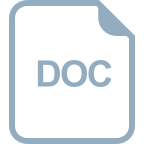