Springboot实现文件上传和下载
时间: 2024-05-10 17:15:23 浏览: 4
Spring Boot 提供了方便的方式来实现文件上传和下载。下面是一个简单的示例:
## 上传文件
### HTML页面
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>文件上传</title>
</head>
<body>
<form method="post" enctype="multipart/form-data" action="/upload">
<input type="file" name="file" />
<br/><br/>
<input type="submit" value="上传" />
</form>
</body>
</html>
```
### Controller
```java
@RestController
public class FileUploadController {
@PostMapping("/upload")
public String upload(@RequestParam("file") MultipartFile file) throws IOException {
// 保存文件
byte[] bytes = file.getBytes();
Path path = Paths.get("uploads/" + file.getOriginalFilename());
Files.write(path, bytes);
return "上传成功!";
}
}
```
## 下载文件
### Controller
```java
@RestController
public class FileDownloadController {
@GetMapping("/download/{fileName}")
public ResponseEntity<Resource> download(@PathVariable String fileName) throws IOException {
// 加载文件资源
Path path = Paths.get("uploads/" + fileName);
Resource resource = new UrlResource(path.toUri());
// 设置响应头
HttpHeaders headers = new HttpHeaders();
headers.add(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=\"" + resource.getFilename() + "\"");
return ResponseEntity.ok()
.headers(headers)
.contentLength(resource.contentLength())
.contentType(MediaType.APPLICATION_OCTET_STREAM)
.body(resource);
}
}
```
### HTML页面
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>文件下载</title>
</head>
<body>
<a href="/download/文件名">下载文件</a>
</body>
</html>
```
以上就是一个简单的文件上传和下载的示例。需要注意的是,这里没有做任何的文件校验和安全性处理,实际开发中需要加强这方面的处理。
相关推荐
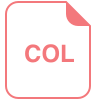
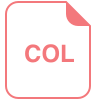
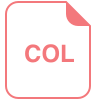
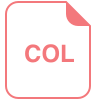
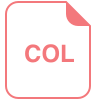









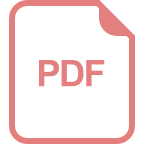