基于java的科学计算器设计
时间: 2023-12-01 19:04:27 浏览: 83
为了设计基于Java的科学计算器,你需要考虑以下几个方面:
1. 用户界面设计:你需要设计一个用户友好的界面,使用户可以方便地输入表达式并查看计算结果。你可以使用Java Swing或JavaFX等GUI工具包来实现用户界面。
2. 表达式解析:你需要将用户输入的表达式解析为可以进行运算的形式。你可以使用逆波兰表达式或语法树来实现表达式解析。
3. 计算功能:你需要实现各种计算功能,例如加、减、乘、除、三角函数、对数函数、指数函数等。可以使用Java Math类中已经提供的函数或自己实现这些函数。
4. 错误处理:你需要考虑如何处理用户输入错误的情况,例如除数为零、输入非法字符等。
5. 单元测试:为了保证计算器的正确性,你需要编写单元测试来测试各种计算功能的正确性。
总之,实现一个基于Java的科学计算器需要涉及多个方面,需要综合考虑用户体验、算法实现、错误处理等方面。
相关问题
基于java的科学计算器设计代码
以下是一个简单的基于Java的科学计算器设计代码,仅供参考:
```
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class ScientificCalculator extends JFrame implements ActionListener {
private JTextField inputField;
private JLabel outputLabel;
public ScientificCalculator() {
setTitle("Scientific Calculator");
setLayout(new FlowLayout());
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
inputField = new JTextField(20);
add(inputField);
JButton calculateButton = new JButton("Calculate");
calculateButton.addActionListener(this);
add(calculateButton);
outputLabel = new JLabel("Result: ");
add(outputLabel);
pack();
setLocationRelativeTo(null); // Center the window on the screen
}
@Override
public void actionPerformed(ActionEvent e) {
String input = inputField.getText();
try {
double result = evaluate(input);
outputLabel.setText("Result: " + result);
} catch (Exception ex) {
outputLabel.setText("Error: " + ex.getMessage());
}
}
private double evaluate(String input) {
// TODO: Implement the expression parsing and evaluation logic here
return 0.0;
}
public static void main(String[] args) {
ScientificCalculator calculator = new ScientificCalculator();
calculator.setVisible(true);
}
}
```
这个代码实现了一个简单的带有文本框、计算按钮和输出标签的GUI界面,当用户点击计算按钮时,程序将会调用 `evaluate` 方法来计算用户输入的表达式,并将结果显示在输出标签中。你需要自己实现 `evaluate` 方法来实现表达式的解析和计算。
Java科学计算器代码
这里提供一个基于Java Swing实现的科学计算器代码:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class ScientificCalculator extends JFrame implements ActionListener {
private JTextField outputField;
private String inputStr = "";
private String operation = "";
private double result = 0.0;
public ScientificCalculator() {
// 设置窗口标题
super("Scientific Calculator");
// 创建面板
JPanel mainPanel = new JPanel();
mainPanel.setLayout(new BorderLayout());
// 创建文本框
outputField = new JTextField("");
outputField.setHorizontalAlignment(JTextField.RIGHT);
outputField.setEditable(false);
mainPanel.add(outputField, BorderLayout.NORTH);
// 创建按钮
JPanel buttonPanel = new JPanel();
buttonPanel.setLayout(new GridLayout(5, 4, 5, 5));
String[] buttonLabels = {
"7", "8", "9", "/",
"4", "5", "6", "*",
"1", "2", "3", "-",
"0", ".", "C", "+",
"sin", "cos", "tan", "="
};
for (int i = 0; i < buttonLabels.length; i++) {
JButton button = new JButton(buttonLabels[i]);
button.addActionListener(this);
buttonPanel.add(button);
}
mainPanel.add(buttonPanel, BorderLayout.CENTER);
// 添加面板到窗口
add(mainPanel);
// 设置窗口大小和位置
setSize(300, 300);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
public void actionPerformed(ActionEvent event) {
String command = event.getActionCommand();
// 处理数字输入
if (command.matches("[0-9.]")) {
inputStr += command;
outputField.setText(inputStr);
}
// 处理运算符输入
else if (command.matches("[/+*-]")) {
if (inputStr.isEmpty()) {
inputStr = Double.toString(result);
}
result = Double.parseDouble(inputStr);
operation = command;
inputStr = "";
}
// 处理三角函数运算
else if (command.matches("(sin|cos|tan)")) {
double value = Double.parseDouble(inputStr);
switch (command) {
case "sin":
value = Math.sin(value);
break;
case "cos":
value = Math.cos(value);
break;
case "tan":
value = Math.tan(value);
break;
}
result = value;
inputStr = "";
outputField.setText(Double.toString(result));
}
// 处理等号运算
else if (command.equals("=")) {
if (inputStr.isEmpty()) {
inputStr = Double.toString(result);
}
double value = Double.parseDouble(inputStr);
switch (operation) {
case "/":
result /= value;
break;
case "*":
result *= value;
break;
case "+":
result += value;
break;
case "-":
result -= value;
break;
}
inputStr = "";
outputField.setText(Double.toString(result));
}
// 处理清空操作
else if (command.equals("C")) {
inputStr = "";
operation = "";
result = 0.0;
outputField.setText("");
}
}
public static void main(String[] args) {
ScientificCalculator calculator = new ScientificCalculator();
calculator.setVisible(true);
}
}
```
这个计算器支持基本的加减乘除运算,以及三角函数的计算。你可以根据自己的需要对代码进行修改和扩展。
阅读全文
相关推荐
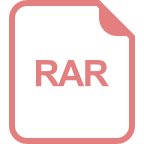
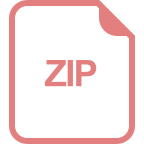
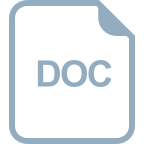
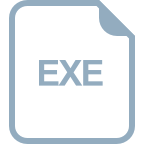
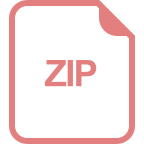
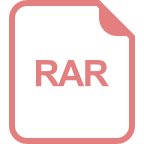
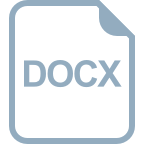
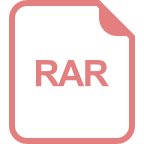
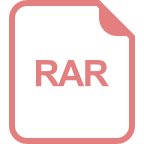
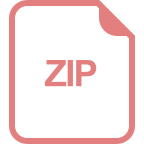
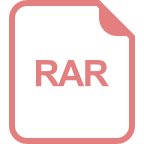
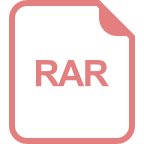
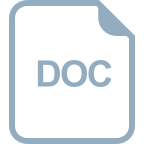