vue3 setattribute
时间: 2024-10-23 16:12:43 浏览: 6
Vue.js 3.x 中的 `setAttribute` 是一个DOM操作方法,用于设置HTML元素的属性值。在JavaScript原生API里,`setAttribute(name, value)`用于给指定元素添加或更新属性,其中`name`是你想要改变的属性名,`value`则是该属性的新值。
在Vue组件中,虽然直接使用`setAttribute`不是最佳实践,因为它违背了组件内部状态管理的理念,通常我们会在模板中使用`v-bind`或简写的`:`绑定动态数据到属性上。例如:
```html
<div v-bind:class="{ active: isActive }">...</div>
```
这是通过`v-bind:class`指令将`isActive`属性的数据映射到`class`属性上,当`isActive`变化时,对应的样式会自动应用。
然而,如果你确实需要在特定场景下手动修改DOM属性,比如响应式劫持某些非Vue管理的属性,可以这样做:
```javascript
this.$el.setAttribute('data-custom', 'someValue');
```
但这并不是常规做法,并可能导致数据流控制的问题。Vue更倾向于数据驱动视图,所以除非有特殊需求,建议尽量避免直接操作DOM。
相关问题
vue利用a标签的setAttribute下载excel文件
可以使用以下代码:
```vue
<template>
<div>
<a href="#" @click="downloadExcel">Download Excel</a>
</div>
</template>
<script>
export default {
methods: {
downloadExcel() {
const blob = new Blob(['Excel file content'], { type: 'application/vnd.ms-excel' });
const url = window.URL.createObjectURL(blob);
const link = document.createElement('a');
link.setAttribute('href', url);
link.setAttribute('download', 'example.xlsx');
link.click();
},
},
};
</script>
```
在`downloadExcel`方法中,我们首先创建一个Blob对象,其中包含要下载的Excel文件的内容和MIME类型。然后使用`window.URL.createObjectURL()`方法生成一个URL,将其设置为a标签的href属性。最后,将a标签的download属性设置为要下载的文件名,并调用`click()`方法以触发下载。
this.$el.querySelector('input').setAttribute('inputmode', 'none') vue3写法
在 Vue3 中,如果你想要通过 `this` 指针操作 DOM 并设置元素属性,可以使用 `ref` 来获取组件内部元素的引用,然后像这样操作:
```html
<!-- 在模板中 -->
<input ref="myInput" />
// 在 script 部分
export default {
setup() {
const myInput = ref(null); // 初始化 ref
// 当组件挂载后,通过 this.$el 获取根元素
onMounted(() => {
// 使用 .value 而不是直接 .querySelector('input') 因为 ref 返回的是具体的 Vue 实例
this.$el.querySelector('input')?.then(inputEl => {
if (inputEl) {
// 设置 inputmode 属性
inputEl.setAttribute('inputmode', 'none');
}
});
});
return { myInput };
},
};
```
上述代码首先声明了一个名为 `myInput` 的 `ref`,然后在组件挂载时查询输入框并设置其 `inputmode` 属性。注意这里使用了箭头函数和可选链操作符 (`?.`) 防止在 `inputEl` 未找到的情况下抛出错误。
阅读全文
相关推荐
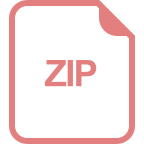
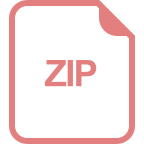
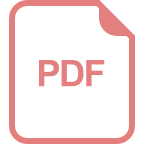













