使用c语言为某个单位建立一个员工通讯录管理系统,可以方便查询每一个员工的电话与地址。设计哈希表存储,设计并实现通讯录查找系统。要求以用户名位关键字,使用哈希函数采用除留余数法,解决冲突采用二次探测再散列法
时间: 2024-09-29 09:07:37 浏览: 52
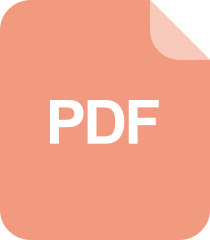
C语言基于哈希表实现通讯录

在C语言中创建一个员工通讯录管理系统,我们可以利用哈希表数据结构来高效地存储和查询每个员工的信息。首先,我们需要设计哈希表,这通常是一个数组,其大小由我们选择的哈希函数确定,这里选择的是除留余数法,也称为取模法。
```c
typedef struct Employee {
char username[50];
char phone[15];
char address[100];
} Employee;
#define MAX_EMPLOYEES 100 // 哈希表最大容量
Employee* employeeHashTable[MAX_EMPLOYEES]; // 哈希表
// 哈希函数,使用取模法计算键值
int hashFunction(char* username) {
int hashValue = 0;
for (int i = 0; username[i] != '\0'; ++i) {
hashValue += username[i];
}
return hashValue % MAX_EMPLOYEES;
}
// 插入员工信息
void insertEmployee(Employee* emp, char* username, char* phone, char* address) {
int index = hashFunction(username);
if (employeeHashTable[index] == NULL) {
employeeHashTable[index] = malloc(sizeof(Employee));
strcpy(employeeHashTable[index]->username, username);
strcpy(employeeHashTable[index]->phone, phone);
strcpy(employeeHashTable[index]->address, address);
} else {
// 冲突处理,使用二次探测再散列
int probeIndex = index;
while (employeeHashTable[probeIndex] != NULL) {
probeIndex = (probeIndex + 1) % MAX_EMPLOYEES;
if (employeeHashTable[probeIndex] == NULL) {
break;
}
}
employeeHashTable[probeIndex] = malloc(sizeof(Employee));
strcpy(employeeHashTable[probeIndex]->username, username);
strcpy(employeeHashTable[probeIndex]->phone, phone);
strcpy(employeeHashTable[probeIndex]->address, address);
}
}
// 查找员工信息
Employee* searchEmployee(char* username) {
int index = hashFunction(username);
while (employeeHashTable[index] != NULL) {
if (strcmp(employeeHashTable[index]->username, username) == 0) {
return employeeHashTable[index];
}
index = (index + 1) % MAX_EMPLOYEES; // 二次探测
}
return NULL; // 没找到对应员工
}
```
阅读全文
相关推荐
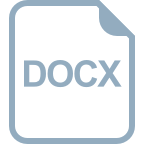
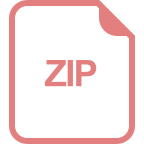
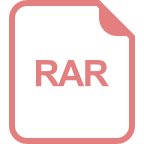
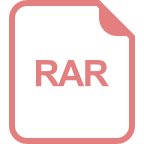
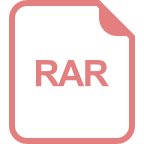
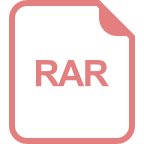
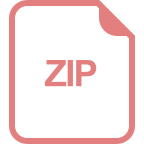
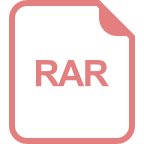
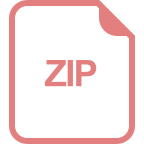
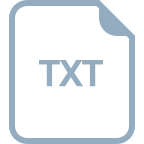
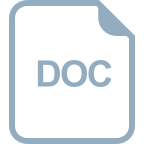
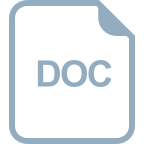

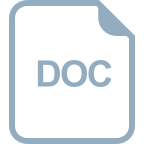

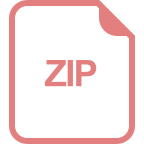