用c++语言通过使用散列表实现一个拼写检查程序
时间: 2024-10-21 07:13:22 浏览: 21
在C++中,我们可以使用散列表(也称为哈希表或关联数组)来实现一个基本的拼写检查程序。散列表提供常数时间复杂度的查找、插入和删除操作,这对于快速查找单词是否在词典中非常有用。以下是简单的步骤:
1. **创建散列表结构**:
首先,我们需要定义一个`std::unordered_set<std::string>`,它是一个无序的键值对集合,键是单词,值可以忽略。
```cpp
#include <iostream>
#include <unordered_set>
std::unordered_set<std::string> dictionary;
```
2. **填充词典**:
将正确的拼写单词添加到散列表中。这个过程通常是读取文件或数据库,将每个单词转换为小写并插入。
```cpp
void loadDictionary(const std::string& filename) {
std::ifstream file(filename);
if (file.is_open()) {
std::string word;
while (file >> word) {
// 转换为小写并添加到字典
dictionary.insert(word.lower());
}
file.close();
} else {
std::cerr << "无法打开文件" << std::endl;
}
}
```
3. **拼写检查函数**:
接收用户输入的单词,检查它是否存在于字典中。如果存在,返回true,反之则返回false。
```cpp
bool isCorrectSpelling(const std::string& input) {
// 先转为小写以进行一致的比较
std::string lowerInput = input.lower();
return dictionary.find(lowerInput) != dictionary.end();
}
```
4. **主程序示例**:
在主函数中,你可以读取用户输入,然后检查拼写。
```cpp
int main() {
loadDictionary("dictionary.txt");
std::string userWord;
std::cout << "请输入单词: ";
std::getline(std::cin, userWord);
bool correct = isCorrectSpelling(userWord);
if (correct) {
std::cout << "拼写正确!" << std::endl;
} else {
std::cout << "拼写错误,建议查阅词典." << std::endl;
}
return 0;
}
```
阅读全文
相关推荐






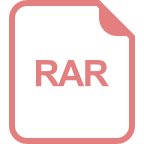

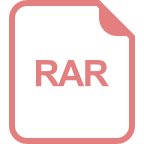





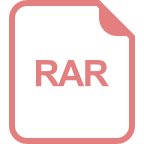

