springboot物资库存的增删改查示例代码
时间: 2023-11-08 11:04:32 浏览: 72
以下是一个简单的Spring Boot示例代码,演示物资库存的增删改查功能:
首先,创建一个名为Goods的实体类,表示物资:
```java
@Entity
@Table(name = "goods")
public class Goods {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private Integer quantity;
// 构造函数、Getter和Setter省略...
}
```
然后,创建一个名为GoodsRepository的接口,用于定义数据库操作:
```java
@Repository
public interface GoodsRepository extends JpaRepository<Goods, Long> {
}
```
接下来,创建一个名为GoodsService的类,用于封装业务逻辑:
```java
@Service
public class GoodsService {
@Autowired
private GoodsRepository goodsRepository;
public List<Goods> getAllGoods() {
return goodsRepository.findAll();
}
public Goods getGoodsById(Long id) {
return goodsRepository.findById(id).orElse(null);
}
public Goods createGoods(Goods goods) {
return goodsRepository.save(goods);
}
public Goods updateGoods(Long id, Goods updatedGoods) {
Goods existingGoods = goodsRepository.findById(id).orElse(null);
if (existingGoods != null) {
existingGoods.setName(updatedGoods.getName());
existingGoods.setQuantity(updatedGoods.getQuantity());
return goodsRepository.save(existingGoods);
}
return null;
}
public void deleteGoods(Long id) {
goodsRepository.deleteById(id);
}
}
```
最后,在控制器类中使用GoodsService来处理HTTP请求:
```java
@RestController
@RequestMapping("/goods")
public class GoodsController {
@Autowired
private GoodsService goodsService;
@GetMapping
public List<Goods> getAllGoods() {
return goodsService.getAllGoods();
}
@GetMapping("/{id}")
public Goods getGoodsById(@PathVariable Long id) {
return goodsService.getGoodsById(id);
}
@PostMapping
public Goods createGoods(@RequestBody Goods goods) {
return goodsService.createGoods(goods);
}
@PutMapping("/{id}")
public Goods updateGoods(@PathVariable Long id, @RequestBody Goods updatedGoods) {
return goodsService.updateGoods(id, updatedGoods);
}
@DeleteMapping("/{id}")
public void deleteGoods(@PathVariable Long id) {
goodsService.deleteGoods(id);
}
}
```
这是一个简单的示例代码,演示了如何使用Spring Boot实现物资库存的增删改查功能。你可以根据实际需求进行修改和扩展。
相关推荐
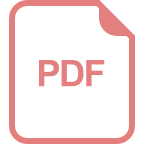
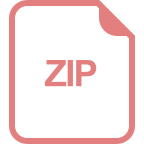
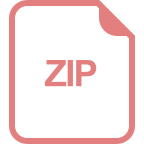














