通过顺序存储结构与链式存储结构分别实现括号匹配,并且可以通过菜单选项选择的c语言代码
时间: 2024-11-09 22:26:14 浏览: 20
在C语言中,我们通常会使用队列数据结构来实现括号匹配,因为队列遵循先进先出(FIFO)的原则,可以方便地检查左括号是否配对右括号。这里我会给出两个示例,一个是基于顺序存储(数组)的实现,另一个是基于链式存储(动态内存分配)的实现。
**基于数组的顺序存储实现 (栈):**
```c
#include <stdio.h>
#include <stdbool.h>
bool is_balanced(char brackets[]) {
int stack[100], top = -1;
for (int i = 0; brackets[i] != '\0'; ++i) {
if (brackets[i] == '(' || brackets[i] == '{' || brackets[i] == '[') {
stack[++top] = brackets[i];
} else if (stack[top] != '\0') {
if ((brackets[i] == ')' && stack[top] != '(') ||
(brackets[i] == '}' && stack[top] != '{') ||
(brackets[i] == ']' && stack[top] != '[')) {
return false;
}
stack[top--] = '\0';
} else {
return false; // 如果遇到空括号,说明之前已有未配对的
}
}
return top == -1; // 如果栈为空,则所有括号已匹配
}
int main() {
char input[] = "(({}))";
if (is_balanced(input))
printf("括号匹配\n");
else
printf("括号不匹配\n");
return 0;
}
```
**基于链表的链式存储实现 (递归节点):**
```c
#include <stdbool.h>
typedef struct Node {
char data;
struct Node* next;
} StackNode;
StackNode* push(StackNode** head, char data) {
StackNode* newNode = (StackNode*)malloc(sizeof(StackNode));
newNode->data = data;
newNode->next = *head;
*head = newNode;
return *head;
}
bool isBalancedRec(StackNode* head) {
if (!head)
return true;
if (head->data == ')')
return !isBalancedRec(head->next) || head->next->data == '(';
else if (head->data == ']')
return !isBalancedRec(head->next) || head->next->data == '[';
else if (head->data == '}')
return !isBalancedRec(head->next) || head->next->data == '{';
else
return isBalancedRec(head->next);
}
int main() {
StackNode* head = NULL;
char input[] = "({[()]})";
if (isBalancedRec(push(&head, input[0])))
printf("括号匹配\n");
else
printf("括号不匹配\n");
return 0;
}
```
阅读全文
相关推荐
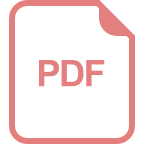
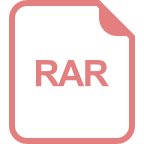
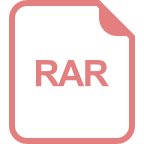
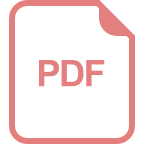
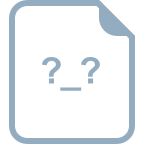
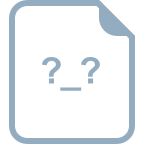
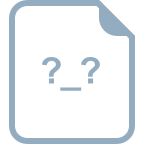
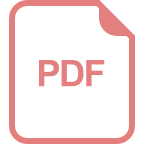