使用位运算计算两个输入数字的和C语言
时间: 2024-10-03 14:02:18 浏览: 35
在C语言中,你可以使用位运算来计算两个整数的和,特别是当涉及到二进制表示时,这种方法通常用于性能优化,尤其是在处理大量数据时。例如,对于无符号整型(如`unsigned int`),你可以利用按位与(`&`)、按位异或(`^`)以及左移(`<<`)操作来进行加法。
下面是一个简单的例子,展示了如何使用位运算实现两个无符号整数的加法:
```c
#include <stdio.h>
unsigned int addWithoutCarry(unsigned int a, unsigned int b) {
// XOR (exclusive or) the bits to get the sum without carry
unsigned int sum = a ^ b;
// AND then left shift one of the numbers by one bit to get the carry
// Note that if there's no carry in the least significant bit, b will be zero after shifting, so we use a
unsigned int carry = (a & b) << 1; // For 32-bit integers, it's typically a & (b << 1)
// Repeat the process until there's no more carry
while (carry != 0) {
a = sum;
b = carry;
sum = a ^ b;
carry = (a & b) << 1;
}
return sum;
}
int main() {
unsigned int x = 5, y = 7;
printf("Sum using bit operations: %u\n", addWithoutCarry(x, y));
return 0;
}
```
阅读全文
相关推荐
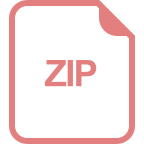
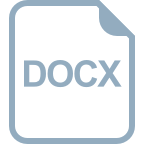
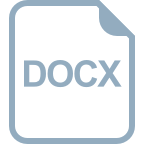
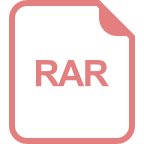
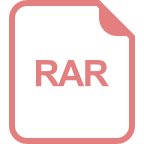
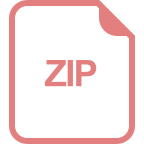
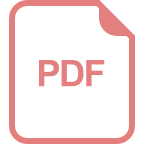
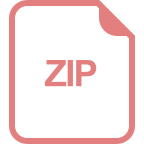
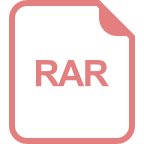
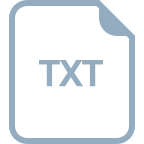
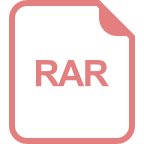
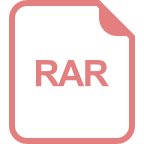
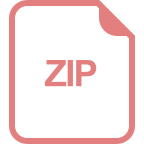
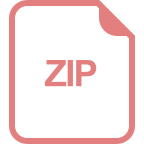
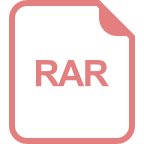
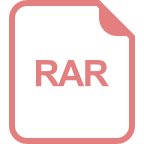
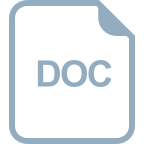
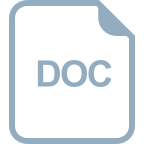
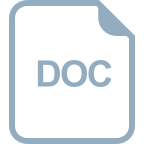