java编写:定义一个类“字符统计”,包含如下方法“数字字符频次统计”“字母字符频次统计”“非数字、非字母等其他字符频次统计”“获取数字频次信息”“获取字母频次信息”“获取非数字、非字母等其他字符频次信息”;包含如下属性“数字字符频次表”“字母字符频次表”“非数字、非字母等其他字符频次表” 定义一个测试类,在测试类中定义个长度不小于200的字符串,要求改字符串中包含数字、英文字母、其他字符。利用字符统计类创建一个统计类对象,分别调用“数字字符频次统计”“字母字符频次统计”“非数字、非字母等其他字符频次统计”方法,对定义的字符串进行统计,统计后,再分别调用“获取数字频次信息”“获取字母频次信息”“获取非数字、非字母等其他字符频次信息”方法,将统计信息打印。
时间: 2023-02-09 08:54:21 浏览: 107
下面是类“字符统计”的示例代码:
```java
class CharacterStatistics {
private int[] digitFrequency;
private int[] letterFrequency;
private int[] otherFrequency;
public void digitFrequencyCount(String str) {
digitFrequency = new int[10];
for (int i = 0; i < str.length(); i++) {
if (Character.isDigit(str.charAt(i))) {
digitFrequency[str.charAt(i) - '0']++;
}
}
}
public void letterFrequencyCount(String str) {
letterFrequency = new int[26];
for (int i = 0; i < str.length(); i++) {
if (Character.isLetter(str.charAt(i))) {
letterFrequency[Character.toLowerCase(str.charAt(i)) - 'a']++;
}
}
}
public void otherFrequencyCount(String str) {
otherFrequency = new int[1];
for (int i = 0; i < str.length(); i++) {
if (!Character.isLetterOrDigit(str.charAt(i))) {
otherFrequency[0]++;
}
}
}
public int[] getDigitFrequency() {
return digitFrequency;
}
public int[] getLetterFrequency() {
return letterFrequency;
}
public int[] getOtherFrequency() {
return otherFrequency;
}
}
```
下面是测试类的示例代码:
```java
public class Test {
public static void main(String[] args) {
String str = "This is a string containing numbers(123), letters(abc) and other characters(#@!).";
CharacterStatistics statistics = new CharacterStatistics();
statistics.digitFrequencyCount(str);
statistics.letterFrequencyCount(str);
statistics.otherFrequencyCount(str);
int[] digitFrequency = statistics.getDigitFrequency();
int[] letterFrequency = statistics.getLetterFrequency();
int[] otherFrequency = statistics.getOtherFrequency();
System.out.println("Digit frequency: " + Arrays.toString(digitFrequency));
System.out.println("Letter frequency: " + Arrays.toString(letterFrequency));
System.out.println("Other frequency: " + Arrays.toString(otherFrequency));
}
}
```
注意:上面代码需要在java1.5及其以上版本运行,需要引入java.util.Arrays
阅读全文
相关推荐














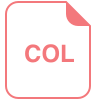
