银行家算法Java实现
时间: 2023-11-14 17:07:14 浏览: 148
银行家算法是一种避免死锁的算法,它通过动态地分配资源来避免进程因争夺资源而产生死锁。下面是银行家算法的Java实现:
```java
import java.util.Scanner;
public class BankerAlgorithm {
private int[][] need; // 需求矩阵
private int[][] allocation; // 分配矩阵
private int[][] max; // 最大需求矩阵
private int[] available; // 可用资源向量
private int processNum; // 进程数
private int resourceNum; // 资源数
public BankerAlgorithm(int[][] need, int[][] allocation, int[][] max, int[] available) {
this.need = need;
this.allocation = allocation;
this.max = max;
this.available = available;
this.processNum = allocation.length;
this.resourceNum = available.length; }
// 判断是否满足需求
private boolean check(int[] work, boolean[] finish, int[] need, int[] allocation) {
for (int i = 0; i < resourceNum; i++) {
if (need[i] > work[i]) {
return false;
}
}
for (int i = 0; i < resourceNum; i++) {
work[i] += allocation[i];
}
finish[processNum] = true;
return true;
}
// 执行银行家算法
public boolean execute() {
boolean[] finish = new boolean[processNum];
int[] work = new int[resourceNum];
for (int i = 0; i < resourceNum; i++) {
work[i] = available[i];
}
int count = 0;
while (count < processNum) {
boolean flag = false;
for (int i = 0; i < processNum; i++) {
if (!finish[i] && check(work, finish, need[i], allocation[i])) {
flag = true;
count++;
}
}
if (!flag) {
return false;
}
}
return true;
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入进程数:");
int processNum = scanner.nextInt();
System.out.print("请输入资源数:");
int resourceNum = scanner.nextInt();
int[][] max = new int[processNum][resourceNum];
int[][] allocation = new int[processNum][resourceNum];
int[][] need = new int[processNum][resourceNum];
int[] available = new int[resourceNum];
System.out.println("请输入最大需求矩阵:");
for (int i = 0; i < processNum; i++) {
for (int j = 0; j < resourceNum; j++) {
max[i][j] = scanner.nextInt();
}
}
System.out.println("请输入分配矩阵:");
for (int i = 0; i < processNum; i++) {
for (int j = 0; j < resourceNum; j++) {
allocation[i][j] = scanner.nextInt();
need[i][j] = max[i][j] - allocation[i][j];
}
}
System.out.println("请输入可用资源向量:");
for (int i = 0; i < resourceNum; i++) {
available[i] = scanner.nextInt();
}
BankerAlgorithm bankerAlgorithm = new BankerAlgorithm(need, allocation, max, available);
if (bankerAlgorithm.execute()) {
System.out.println("系统是安全的!");
} else {
System.out.println("系统是不安全的!");
}
}
}
```
阅读全文
相关推荐

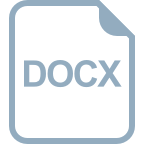
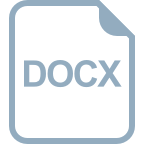
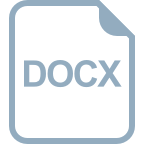
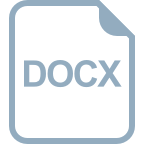
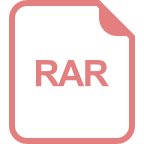
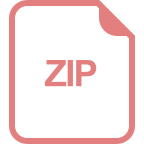
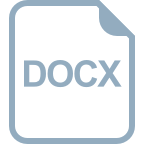
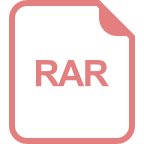
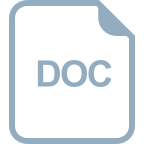
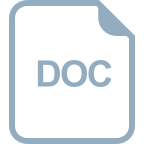


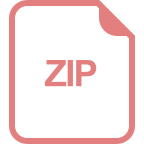
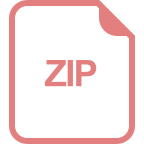
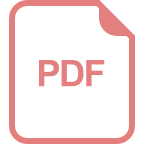
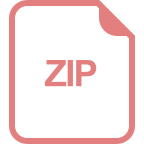