QTableView的下拉控件代理怎么写
时间: 2024-11-28 14:28:18 浏览: 14
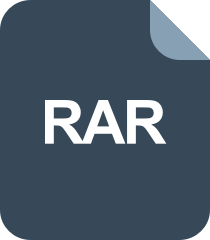
Qt QTableView插入控件,qss设计
在QTableView中实现下拉控件代理通常涉及到`QAbstractItemDelegate`的派生类以及`QStyleOptionViewItem`和`QPainter`的使用。你需要创建一个自定义的代理(例如,`CustomComboBoxDelegate`),并在其中处理单元格的编辑过程,包括显示下拉列表、选择项并更新数据。
以下是一个简单的步骤概述:
1. **继承基础委托**:
```cpp
class CustomComboBoxDelegate : public QAbstractItemDelegate {
//...
};
```
2. **设置样式选项**:
```cpp
void setEditorData(QAbstractItemDelegate::EditorWidget *editor, const QModelIndex &index) override {
QComboBox *comboBox = qobject_cast<QComboBox*>(editor);
comboBox->setModel(yourComboBoxModel); // 设置下拉模型
// 初始化下拉框内容
}
void setModelData(QWidget *editor, QAbstractItemModel *model, const QModelIndex &index) override {
QComboBox *comboBox = qobject_cast<QComboBox*>(editor);
int selectedValue = comboBox->currentIndex();
model->setData(index, selectedValue); // 更新表格中的值
}
```
3. **绘制编辑控制**:
```cpp
void paint(QPainter *painter, const QStyleOptionViewItem &option, const QModelIndex &index) override {
if (index.column() == columnToEdit && option.state & QStyle::State_Enabled) {
painter->save();
// 其他绘画逻辑...
// 显示下拉箭头或图标
QRect rect = option.rect;
QRect arrowRect(rect.right() - 6, rect.center().y(), 8, 8);
style()->drawPrimitive(QStyle::PE_PanelItemViewItemDropIndicator, &arrowRect, painter, option);
painter->restore();
}
QAbstractItemDelegate::paint(this, painter, option, index);
}
```
4. **设置代理给QTableView**:
```cpp
tableView.setItemDelegate(new CustomComboBoxDelegate(tableView));
```
5. **处理事件**:
如果需要处理用户的选择事件,可以在`QTableView`上连接信号和槽,比如 `currentIndexChanged(int)`。
阅读全文
相关推荐
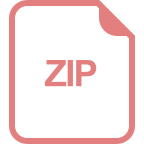
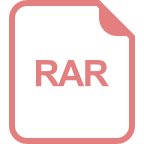
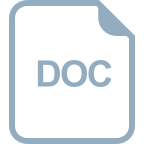
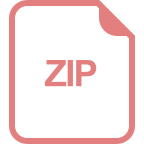
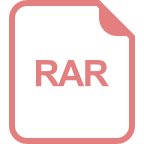
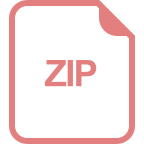
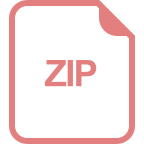
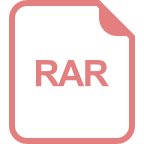
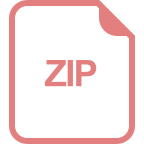
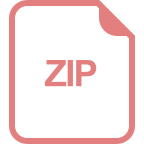
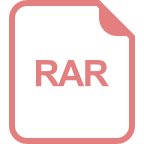
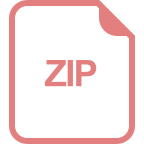
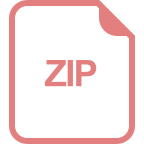
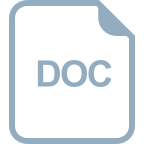
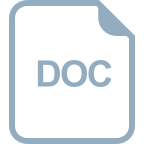
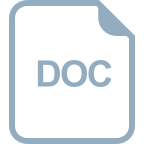
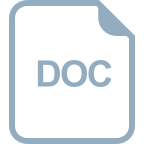
