写一个spring boot vue 用户头像上传代码
时间: 2023-12-02 20:04:54 浏览: 43
好的,以下是一个简单的 Spring Boot + Vue.js 项目中用户头像上传的代码。
前端代码:
```
<template>
<div>
<img :src="imageUrl" :alt="username" width="100">
<form>
<input type="file" @change="onFileChange" accept="image/*">
<button type="button" @click="upload">上传头像</button>
</form>
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
imageUrl: '',
username: 'test'
}
},
methods: {
onFileChange(e) {
const file = e.target.files[0];
this.imageUrl = URL.createObjectURL(file);
this.file = file;
},
upload() {
const formData = new FormData();
formData.append('file', this.file);
formData.append('username', this.username);
axios.post('/api/upload', formData).then(response => {
console.log(response.data);
}).catch(error => {
console.log(error);
});
}
}
}
</script>
```
后端代码:
```
@RestController
@RequestMapping("/api")
public class UserController {
@Autowired
private UserService userService;
@PostMapping(value = "/upload", consumes = MediaType.MULTIPART_FORM_DATA_VALUE)
public ResponseEntity<Object> uploadFile(@RequestParam("file") MultipartFile file,
@RequestParam("username") String username) {
try {
User user = userService.getUserByUsername(username);
if (user != null) {
String fileName = StringUtils.cleanPath(file.getOriginalFilename());
String fileExtension = fileName.substring(fileName.lastIndexOf("."));
String newFileName = UUID.randomUUID().toString() + fileExtension;
String uploadDir = "uploads/";
String filePath = uploadDir + newFileName;
FileUploadUtil.saveFile(uploadDir, newFileName, file);
user.setAvatar(filePath);
userService.saveUser(user);
return ResponseEntity.ok().body("File uploaded successfully!");
} else {
return ResponseEntity.badRequest().body("User not found!");
}
} catch (Exception ex) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body(ex.getMessage());
}
}
}
```
其中,`FileUploadUtil` 是一个工具类,用于保存上传的文件到服务器。`UserService` 是一个服务类,用于处理与用户相关的业务逻辑。`User` 是一个实体类,用于映射数据库中的用户表。
相关推荐
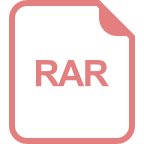
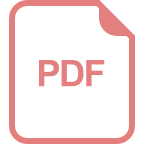
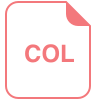
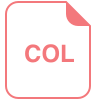
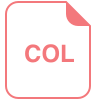
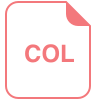
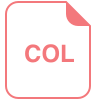









