1.有四个线程,每个线程只打印一个数字,这四个数字分别是 1 2 3 4 ,现在要求你做到四个线程顺序打印 1 2 3 4 ,且每个线程都打印10次.
时间: 2023-11-27 18:51:39 浏览: 68
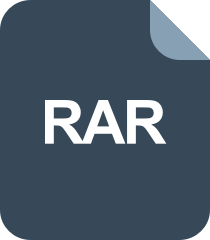
3个线程,根据不同规则打印线程名称
可以使用信号量来实现线程的顺序打印,具体实现如下:
```python
import threading
# 定义信号量
semaphore1 = threading.Semaphore(1)
semaphore2 = threading.Semaphore(0)
semaphore3 = threading.Semaphore(0)
semaphore4 = threading.Semaphore(0)
# 定义线程函数
def thread_func(num, semaphore_current, semaphore_next):
for i in range(10):
# 获取当前信号量
semaphore_current.acquire()
print(num)
# 释放下一个信号量
semaphore_next.release()
# 创建四个线程
thread1 = threading.Thread(target=thread_func, args=(1, semaphore1, semaphore2))
thread2 = threading.Thread(target=thread_func, args=(2, semaphore2, semaphore3))
thread3 = threading.Thread(target=thread_func, args=(3, semaphore3, semaphore4))
thread4 = threading.Thread(target=thread_func, args=(4, semaphore4, semaphore1))
# 启动四个线程
thread1.start()
thread2.start()
thread3.start()
thread4.start()
# 等待四个线程结束
thread1.join()
thread2.join()
thread3.join()
thread4.join()
```
在这个实现中,使用了四个信号量来控制线程的顺序,每个线程在打印完数字后,会释放下一个线程对应的信号量,以便下一个线程能够执行。同时,还需要注意使用 join 方法等待四个线程的结束,以便程序能够正确退出。
阅读全文
相关推荐
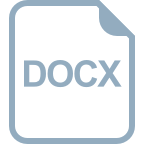
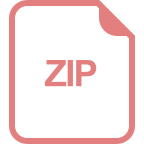
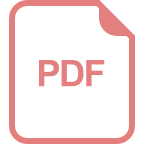
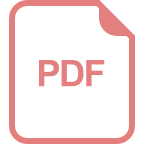
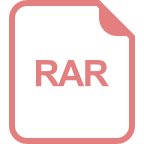
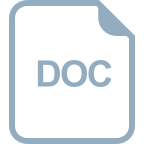
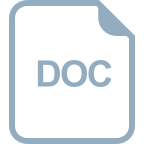
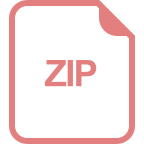
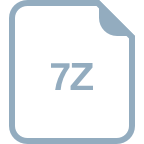
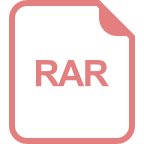
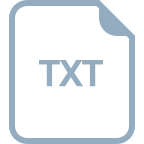
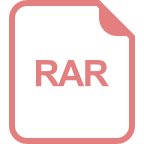
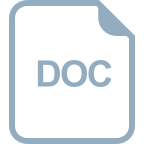
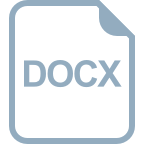
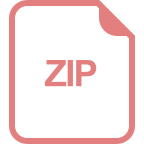
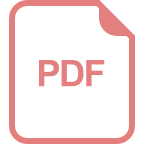