npm install cors --save 本地的服务器怎么跨域支持
时间: 2023-11-15 10:04:12 浏览: 43
安装 `cors` 模块并在 Node.js 中使用它可以让你的本地服务器支持跨域请求。
1. 执行以下命令安装 `cors` 模块:
```bash
npm install cors --save
```
2. 在你的 Node.js 代码中引入和使用 `cors` 模块:
```javascript
const express = require('express');
const cors = require('cors'); // 引入 cors 模块
const app = express();
// 使用 cors 中间件
app.use(cors());
// 在这里添加你的路由和逻辑处理代码
app.listen(8000, () => {
console.log('Server is listening on port 8000');
});
```
这样,你的本地服务器就支持跨域请求了。当然,在生产环境中,你需要根据实际情况配置跨域规则,以保证安全性。
相关问题
配置本地的服务器跨域支持
如果你使用的是 Node.js,可以使用 `cors` 这个包来实现跨域支持。安装 `cors`:
```bash
npm install cors --save
```
然后在你的 Node.js 代码中加入以下代码:
```javascript
const express = require('express')
const cors = require('cors')
const app = express()
app.use(cors())
// 在这里添加你的路由和逻辑处理代码
app.listen(8000, () => {
console.log('Server is listening on port 8000')
})
```
这样就可以支持跨域请求了。注意,在生产环境中,你需要根据实际情况配置跨域规则,以保证安全性。
vue3 element-plus前后端分离解决跨域
在前后端分离的开发模式下,前端和后端是独立的两个系统,前端向后端发送请求时,常常会遇到跨域问题。为了解决这个问题,我们可以在后端进行跨域配置,也可以在前端进行跨域请求。
1. 后端跨域配置
在后端,可以使用 Spring Boot、Node.js、Django 等框架,在配置文件中增加跨域配置,比如在 Spring Boot 中,可以在 application.properties 或 application.yml 中增加以下配置:
```
# 允许所有域名跨域访问
spring.mvc.crossorigin.allowed-origins=*
```
2. 前端跨域请求
在前端,可以使用以下几种方式解决跨域问题:
2.1 代理请求
通过在前端配置代理服务器,将前端的请求转发到后端服务器上,从而避免跨域问题。比如在 Vue.js 中,可以使用 vue-cli-service 的 proxyTable 配置实现代理请求:
```
// vue.config.js
module.exports = {
devServer: {
proxy: {
// 将请求转发到后端服务器上
'/api': {
target: 'http://localhost:8080',
changeOrigin: true,
pathRewrite: {
'^/api': ''
}
}
}
}
}
```
2.2 JSONP 请求
JSONP 请求是一种利用 script 标签的跨域技术,通过在前端页面中动态创建 script 标签,然后在后端返回一个 JSONP 回调函数,前端就可以获取到后端数据了。比如在 Vue.js 中,可以使用 axios-jsonp 库实现 JSONP 请求:
```
// 安装 axios-jsonp 库
npm install axios-jsonp --save
// 使用 axios-jsonp 库发送 JSONP 请求
import axiosJsonp from 'axios-jsonp';
axios({
url: 'http://example.com',
adapter: axiosJsonp
})
.then(response => {
console.log(response);
})
.catch(error => {
console.error(error);
});
```
2.3 CORS 请求
CORS 请求是一种支持跨域的 HTTP 请求,前端可以在请求头中增加 Origin 字段,后端可以在响应头中增加 Access-Control-Allow-Origin 字段,从而允许跨域请求。比如在 Vue.js 中,可以使用 axios 库发送 CORS 请求:
```
// 使用 axios 库发送 CORS 请求
axios({
method: 'get',
url: 'http://example.com',
headers: {
'Origin': 'http://localhost:8080'
}
})
.then(response => {
console.log(response);
})
.catch(error => {
console.error(error);
});
```
以上是解决前后端分离跨域问题的几种方案,具体选择哪种方案需要根据项目实际情况来决定。需要注意的是,在使用代理请求时,应该避免将代理服务器暴露在公网上,以避免安全问题。
相关推荐
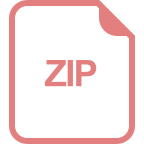












