写个python画兔年年画的程序
时间: 2023-09-19 19:06:27 浏览: 60
### 回答1:
```python
# 画兔年年画程序
from turtle import *
# 设置画笔颜色和粗细
pencolor("brown")
pensize(5)
# 兔头部
circle(30)
# 兔耳朵
penup()
setpos(-25, 40)
pendown()
circle(15)
penup()
setpos(25, 40)
pendown()
circle(15)
# 兔眼睛
penup()
setpos(-10, 55)
pendown()
dot(10)
penup()
setpos(10, 55)
pendown()
dot(10)
# 兔鼻子
penup()
setpos(0, 45)
pendown()
dot(5)
# 兔身体
penup()
setpos(0, -30)
pendown()
circle(50)
# 兔尾巴
penup()
setpos(0, -50)
pendown()
pensize(10)
right(60)
forward(30)
right(120)
forward(30)
# 结束
done()
```
这是一个用Python的turtle库画兔年年画的程序。代码中会用到一些turtle库中的函数,如circle()画圆,dot()画点,setpos()设置画笔位置等。运行后会弹出一个窗口,显示画好的兔年年画。
### 回答2:
下面是一个用Python编写的画兔年年画的程序:
import turtle
# 设置画布大小
turtle.setup(800, 600)
# 配置画笔
turtle.pensize(5)
turtle.pencolor("red")
# 绘制“兔”字
turtle.penup()
turtle.goto(-100, 0)
turtle.pendown()
turtle.seth(-30)
turtle.circle(80, 240)
turtle.seth(90)
turtle.fd(160)
turtle.penup()
turtle.goto(40, -40)
turtle.pendown()
turtle.circle(40, 180)
turtle.penup()
turtle.goto(40, 40)
turtle.pendown()
turtle.circle(40, -180)
# 绘制“年”字
turtle.penup()
turtle.goto(120, 0)
turtle.pendown()
turtle.seth(-90)
turtle.circle(80, 180)
turtle.penup()
turtle.goto(120, 0)
turtle.pendown()
turtle.seth(0)
turtle.fd(160)
turtle.done()
这是一个使用turtle库绘制兔年年画的程序。首先,我们使用turtle.setup()函数来设置画布的大小为800像素宽、600像素高。
然后,我们使用turtle.pensize()函数设置画笔的粗细为5像素,使用turtle.pencolor()函数设置画笔的颜色为红色。
接下来,我们使用turtle的前往和下笔操作来绘制“兔”字。我们先将画笔提起,然后将其移动到起始位置(-100, 0),再放下画笔。使用turtle.seth()函数设置画笔的初始方向为-30度,然后使用turtle.circle()函数绘制一段弧线,形成“兔”字的第一个部分。接着,我们将画笔的方向调整为90度,使用turtle.fd()函数向前移动一定距离,绘制“兔”字的第二个部分。最后,我们将画笔提起,移动到绘制下一个字的起始位置。
之后,我们绘制“年”字。与绘制“兔”字的过程类似,我们使用turtle.penup()函数将画笔提起,然后使用turtle.goto()函数定位到绘制起始点(120, 0)。接着,放下画笔,调整画笔方向和移动距离,使用turtle.circle()和turtle.fd()函数来绘制“年”字。
最后,使用turtle.done()函数来保持图形窗口显示,直到手动关闭。
### 回答3:
下面是使用Python编写的画兔年年画的程序:
```python
import turtle
# 设置画布大小和背景颜色
turtle.setup(600, 600)
turtle.bgcolor('pink')
# 画兔耳朵
def draw_ears():
turtle.penup()
turtle.goto(-80, 0)
turtle.pendown()
turtle.setheading(60)
for _ in range(2):
turtle.circle(80, 120)
turtle.left(120)
# 画兔子的脸
def draw_face():
turtle.penup()
turtle.goto(0, -100)
turtle.pendown()
turtle.setheading(0)
turtle.circle(100)
# 画兔子的眼睛
def draw_eyes():
turtle.penup()
turtle.goto(-20, 20)
turtle.pendown()
turtle.fillcolor('white')
turtle.begin_fill()
turtle.circle(15)
turtle.end_fill()
turtle.penup()
turtle.goto(20, 20)
turtle.pendown()
turtle.fillcolor('white')
turtle.begin_fill()
turtle.circle(15)
turtle.end_fill()
turtle.penup()
turtle.goto(-22, 24)
turtle.pendown()
turtle.fillcolor('black')
turtle.begin_fill()
turtle.circle(8)
turtle.end_fill()
turtle.penup()
turtle.goto(18, 24)
turtle.pendown()
turtle.fillcolor('black')
turtle.begin_fill()
turtle.circle(8)
turtle.end_fill()
# 画兔子的嘴巴
def draw_mouth():
turtle.penup()
turtle.goto(0, -10)
turtle.pendown()
turtle.setheading(-60)
turtle.circle(50, 120)
turtle.penup()
turtle.setheading(0)
turtle.goto(0, -10)
turtle.pendown()
turtle.setheading(-120)
turtle.circle(-50, 120)
# 画兔子的牙齿
def draw_teeth():
turtle.penup()
turtle.goto(-5, -30)
turtle.pendown()
turtle.setheading(0)
turtle.fillcolor('white')
turtle.begin_fill()
for _ in range(2):
turtle.forward(20)
turtle.right(90)
turtle.forward(10)
turtle.right(90)
turtle.end_fill()
# 画兔子的红鼻子
def draw_nose():
turtle.penup()
turtle.goto(0, 10)
turtle.pendown()
turtle.fillcolor('red')
turtle.begin_fill()
turtle.circle(10)
turtle.end_fill()
# 画兔子的胡须
def draw_whiskers():
turtle.penup()
turtle.goto(-20, 0)
turtle.pendown()
turtle.setheading(-30)
turtle.forward(40)
turtle.penup()
turtle.goto(-20, -5)
turtle.pendown()
turtle.setheading(-30)
turtle.forward(40)
turtle.penup()
turtle.goto(-20, -10)
turtle.pendown()
turtle.setheading(-30)
turtle.forward(40)
turtle.penup()
turtle.goto(20, 0)
turtle.pendown()
turtle.setheading(210)
turtle.forward(40)
turtle.penup()
turtle.goto(20, -5)
turtle.pendown()
turtle.setheading(210)
turtle.forward(40)
turtle.penup()
turtle.goto(20, -10)
turtle.pendown()
turtle.setheading(210)
turtle.forward(40)
# 画兔子的身体
def draw_body():
turtle.penup()
turtle.goto(0, -180)
turtle.pendown()
turtle.setheading(-120)
turtle.circle(180, 120)
turtle.left(60)
turtle.circle(180, 120)
# 画兔子的手
def draw_hands():
turtle.penup()
turtle.goto(-180, -220)
turtle.pendown()
turtle.fillcolor('white')
turtle.begin_fill()
turtle.circle(30)
turtle.end_fill()
turtle.penup()
turtle.goto(180, -220)
turtle.pendown()
turtle.fillcolor('white')
turtle.begin_fill()
turtle.circle(30)
turtle.end_fill()
# 画兔子的脚
def draw_feet():
turtle.penup()
turtle.goto(-100, -350)
turtle.pendown()
turtle.setheading(-90)
turtle.color('white')
turtle.fillcolor('white')
turtle.begin_fill()
turtle.forward(60)
turtle.circle(30, 180)
turtle.forward(60)
turtle.left(90)
turtle.forward(160)
turtle.right(90)
turtle.circle(30, 180)
turtle.forward(60)
turtle.end_fill()
turtle.penup()
turtle.goto(100, -350)
turtle.pendown()
turtle.setheading(-90)
turtle.color('white')
turtle.fillcolor('white')
turtle.begin_fill()
turtle.forward(60)
turtle.circle(30, 180)
turtle.forward(60)
turtle.left(90)
turtle.forward(160)
turtle.right(90)
turtle.circle(30, 180)
turtle.forward(60)
turtle.end_fill()
# 画兔子的尾巴
def draw_tail():
turtle.penup()
turtle.goto(200, -250)
turtle.pendown()
turtle.seth(0)
turtle.color('white')
turtle.fillcolor('white')
turtle.begin_fill()
turtle.circle(30, 360)
turtle.end_fill()
if __name__ == '__main__':
draw_ears()
draw_face()
draw_eyes()
draw_mouth()
draw_teeth()
draw_nose()
draw_whiskers()
draw_body()
draw_hands()
draw_feet()
draw_tail()
turtle.done()
```
运行上述代码后,即可绘制出一个兔年年画。这个程序使用了Python的turtle库来进行图形绘制,通过一系列的绘图函数来绘制兔子的各个部分,从耳朵、脸、眼睛、嘴巴、牙齿、鼻子、胡须、身体、手、脚、尾巴等等,最后使用`turtle.done()`显示出绘制的结果。
相关推荐
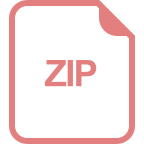
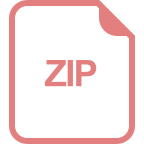














