1,2题要怎么写?给我代码
时间: 2024-12-13 15:25:48 浏览: 3
根据你提供的实验报告内容,以下是第1题和第2题的Python代码实现:
### 第1题:使用Matplotlib绘制柱形图
```python
import matplotlib.pyplot as plt
from faker import Faker
# 初始化Faker对象
fake = Faker()
# 生成数据
x = [fake.word() for _ in range(10)]
y_a = [fake.random_int(min=0, max=100) for _ in range(10)]
y_b = [fake.random_int(min=0, max=100) for _ in range(10)]
# 创建图形
fig, ax = plt.subplots()
# 绘制柱形图
bar_width = 0.35
index = range(len(x))
bars1 = ax.bar(index, y_a, bar_width, label='y_a')
bars2 = ax.bar([i + bar_width for i in index], y_b, bar_width, label='y_b')
# 添加标题和标签
ax.set_title('柱形图示例')
ax.set_xlabel('x轴数据')
ax.set_ylabel('y轴数据')
# 设置x轴刻度
ax.set_xticks([i + bar_width / 2 for i in index])
ax.set_xticklabels(x)
# 添加图例
ax.legend()
# 添加注释文本
for i, v in enumerate(y_a):
ax.text(i, v + 3, str(v), ha='center', va='bottom')
for i, v in enumerate(y_b):
ax.text(i + bar_width, v + 3, str(v), ha='center', va='bottom')
# 显示图形
plt.show()
```
### 第2题:使用Pyecharts绘制多种图表
#### 安装Pyecharts
首先需要安装`pyecharts`库,可以使用以下命令进行安装:
```bash
pip install pyecharts
```
#### 绘制柱形图、词云图、气泡图和圆环图
```python
from pyecharts.charts import Bar, WordCloud, Scatter, Pie
from pyecharts import options as opts
from faker import Faker
# 初始化Faker对象
fake = Faker()
# 生成数据
x = [fake.word() for _ in range(10)]
y_a = [fake.random_int(min=0, max=100) for _ in range(10)]
y_b = [fake.random_int(min=0, max=100) for _ in range(10)]
word_data = [(fake.word(), fake.random_int(min=0, max=100)) for _ in range(50)]
bubble_x = [fake.random_int(min=0, max=100) for _ in range(50)]
bubble_y = [fake.random_int(min=0, max=100) for _ in range(50)]
bubble_size = [fake.random_int(min=10, max=50) for _ in range(50)]
ring_data = [(fake.word(), fake.random_int(min=0, max=100)) for _ in range(5)]
# 绘制柱形图
bar = (
Bar()
.add_xaxis(x)
.add_yaxis("y_a", y_a)
.add_yaxis("y_b", y_b)
.set_global_opts(title_opts=opts.TitleOpts(title="柱形图"))
)
bar.render("bar_chart.html")
# 绘制词云图
wordcloud = (
WordCloud()
.add("", word_data, word_size_range=[20, 100])
.set_global_opts(title_opts=opts.TitleOpts(title="词云图"))
)
wordcloud.render("wordcloud_chart.html")
# 绘制气泡图
scatter = (
Scatter()
.add_xaxis(bubble_x)
.add_yaxis(
"",
bubble_y,
symbol_size=bubble_size,
label_opts=opts.LabelOpts(is_show=False),
)
.set_global_opts(
title_opts=opts.TitleOpts(title="气泡图"),
xaxis_opts=opts.AxisOpts(name="X轴数据"),
yaxis_opts=opts.AxisOpts(name="Y轴数据"),
)
)
scatter.render("scatter_chart.html")
# 绘制圆环图
pie = (
Pie()
.add(
"",
ring_data,
radius=["40%", "75%"],
label_opts=opts.LabelOpts(formatter="{b}: {c} ({d}%)"),
)
.set_global_opts(title_opts=opts.TitleOpts(title="圆环图"))
)
pie.render("ring_chart.html")
```
以上代码分别实现了第1题和第2题的要求。你可以运行这些代码来生成相应的图表,并保存为HTML文件。希望这对你有帮助!
阅读全文
相关推荐
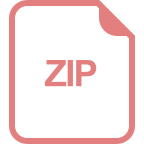
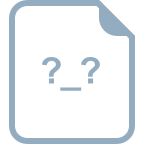
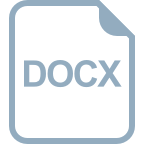
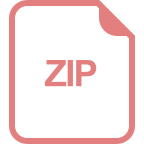
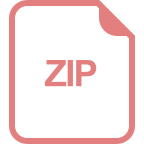
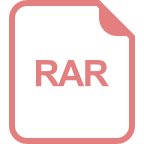
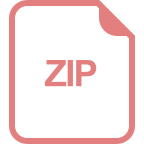
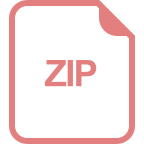
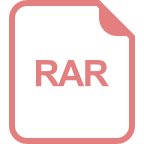
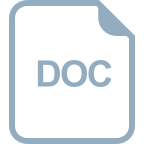
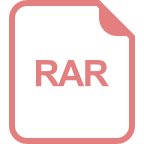
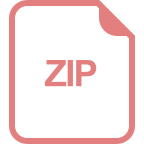
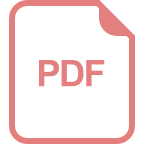
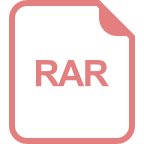
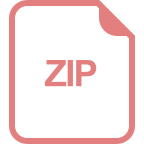
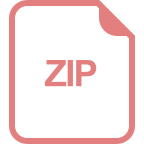
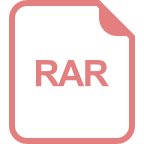
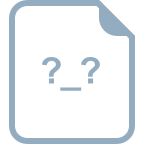