C#发送HttpClient的PostAsync怎么忽略证书
时间: 2024-05-18 14:18:45 浏览: 68
您可以通过创建一个自定义的`HttpClientHandler`,在其中配置忽略证书验证,然后将该`HttpClientHandler`传递给`HttpClient`的构造函数。以下是一个示例代码:
```csharp
// 创建一个忽略证书验证的HttpClientHandler
var handler = new HttpClientHandler()
{
ServerCertificateCustomValidationCallback = HttpClientHandler.DangerousAcceptAnyServerCertificateValidator
};
// 创建一个HttpClient,传入上面创建的handler
var client = new HttpClient(handler);
// 发送一个POST请求
var response = await client.PostAsync("https://example.com/api/endpoint", new StringContent("requestBody"));
// 处理响应
var responseBody = await response.Content.ReadAsStringAsync();
```
需要注意的是,忽略证书验证会存在安全风险,应该谨慎使用。建议在测试或开发阶段使用,生产环境下应该使用有效的证书。
阅读全文
相关推荐
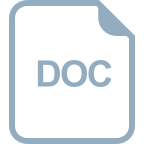
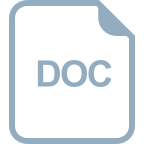
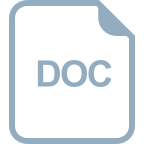
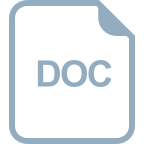
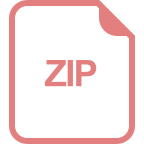
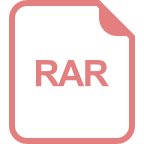
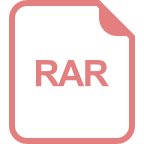
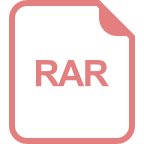
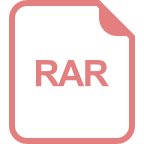
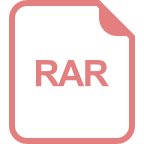
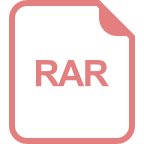
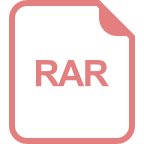
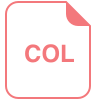
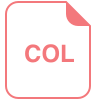
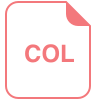
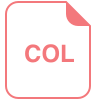
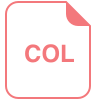
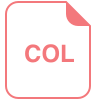
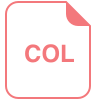