编写一个函数,实现链式存储方式下字符串的replace(S,T1,T2)运算,c语言
时间: 2024-11-20 21:55:41 浏览: 38
在C语言中,链表是一种数据结构,不适合直接用于字符串替换操作,因为链表通常用于动态内存管理,而字符串通常是通过数组或字符指针连续存储的。不过,如果要用链表模拟字符串,我们可以创建一个节点结构来表示每个字符,并链接它们形成一个链表。下面是一个简单的例子,展示了如何实现一个链式存储的单字符节点,以及一个`replace`函数的基本思路。这个例子假设我们有一个自定义的链表节点结构`ListNode`和一个链表表示字符串的头结点`ListNode* strHead`。
```c
typedef struct ListNode {
char data;
struct ListNode* next;
} ListNode;
// 初始化链表表示的空字符串
ListNode* createEmptyString() {
return (ListNode*)malloc(sizeof(ListNode));
}
// 插入字符到链表尾部
void insertCharToList(ListNode** head, char c) {
ListNode* newNode = createEmptyString();
newNode->data = c;
newNode->next = *head;
*head = newNode;
}
// 模拟replace操作,仅支持相邻字符替换
void replaceChars(ListNode** head, char oldChar, char newChar) {
ListNode* current = *head;
while (current != NULL) {
if (current->data == oldChar) {
current->data = newChar;
}
current = current->next;
}
}
// 示例:将链表表示的"hello world"中所有'o'替换成'e'
void mainReplaceExample(char oldChar, char newChar) {
ListNode* strHead = createEmptyString(); // 创建初始链表头
insertCharToList(&strHead, 'h');
insertCharToList(&strHead, 'e');
insertCharToList(&strHead, 'l');
insertCharToList(&strHead, 'l');
insertCharToList(&strHead, 'o');
insertCharToList(&strHead, ' ');
insertCharToList(&strHead, 'w');
insertCharToList(&strHead, 'o');
insertCharToList(&strHead, 'r');
insertCharToList(&strHead, 'l');
insertCharToList(&strHead, 'd');
replaceChars(&strHead, oldChar, newChar); // 替换'o'为'e'
// 打印链表表示的新字符串
// 这里只是一个演示,实际应用需要一个遍历打印链表的完整函数
printLinkedList(strHead);
}
// 辅助函数:打印链表
void printLinkedList(ListNode* head) {
while (head != NULL) {
printf("%c", head->data);
head = head->next;
}
}
```
注意:这个示例非常基础,实际应用中,为了处理更复杂的替换需求(如非相邻字符替换),你可能需要维护额外的数据结构,比如使用两个指针分别指向当前字符和旧字符的位置。
阅读全文
相关推荐
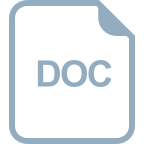
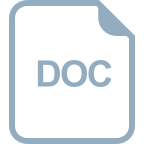
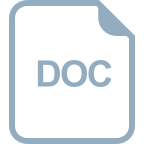








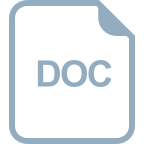
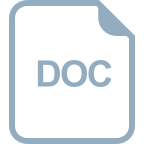
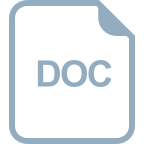
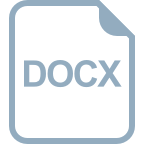