stack在python中的用法
时间: 2023-10-22 08:05:25 浏览: 45
在 Python 中,stack 可以通过列表来实现。stack 是一种后进先出(LIFO)的数据结构,可以用来存储和访问数据。
以下是一些 stack 在 Python 中的常见用法:
1. 创建一个 stack:
```
stack = []
```
2. 将一个元素添加到 stack 中:
```
stack.append(element)
```
3. 从 stack 中删除一个元素:
```
stack.pop()
```
4. 检查 stack 是否为空:
```
if not stack:
print("Stack is empty")
```
5. 获取 stack 的长度:
```
length = len(stack)
```
6. 获取 stack 中的最后一个元素(不删除):
```
last_element = stack[-1]
```
7. 清空 stack:
```
stack.clear()
```
相关问题
python中stack的方法
Python中可以使用列表来实现栈的功能,下面是一些常用的栈方法:
1. push(element): 将元素压入栈顶,即向列表末尾添加元素。
2. pop(): 弹出栈顶元素,并返回该元素。如果栈为空,则会引发 IndexError 异常。
3. peek(): 返回栈顶元素,但不删除它。如果栈为空,则会引发 IndexError 异常。
4. is_empty(): 检查栈是否为空,如果栈为空则返回 True,否则返回 False。
5. size(): 返回栈中元素的数量。
下面是一个示例代码:
```python
class Stack:
def __init__(self):
self.stack = []
def push(self, element):
self.stack.append(element)
def pop(self):
if not self.is_empty():
return self.stack.pop()
else:
raise IndexError("Stack is empty")
def peek(self):
if not self.is_empty():
return self.stack[-1]
else:
raise IndexError("Stack is empty")
def is_empty(self):
return len(self.stack) == 0
def size(self):
return len(self.stack)
# 示例用法
stack = Stack()
stack.push(1)
stack.push(2)
stack.push(3)
print(stack.pop()) # 输出: 3
print(stack.peek()) # 输出: 2
print(stack.is_empty()) # 输出: False
print(stack.size()) # 输出: 2
```
这只是一个简单的示例,你可以根据实际需求进行修改和扩展。
plt中stack plot的用法
stack plot是一种用于显示多个数据系列的堆叠区域图。在matplotlib中,可以使用`stackplot()`函数来创建堆叠区域图。下面是一个简单的例子:
```python
import matplotlib.pyplot as plt
# 数据
x = [1, 2, 3, 4, 5]
y1 = [1, 2, 4, 3, 1]
y2 = [1, 3, 2, 2, 3]
y3 = [1, 1, 2, 4, 4]
# 绘制堆叠区域图
plt.stackplot(x, y1, y2, y3, labels=['y1', 'y2', 'y3'])
# 添加图例
plt.legend(loc='upper left')
# 显示图形
plt.show()
```
在这个例子中,我们创建了三个数据系列y1、y2和y3,并使用`stackplot()`函数将它们绘制成堆叠区域图。我们还使用`labels`参数为每个数据系列添加标签,并使用`legend()`函数添加图例。最后,我们使用`show()`函数显示图形。
相关推荐
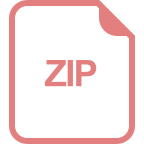
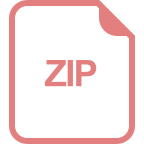
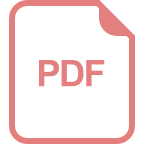












