代码Java+sqlserver的商品信息管理系统,拥有增删改查的功能代码
时间: 2024-03-17 19:43:03 浏览: 68
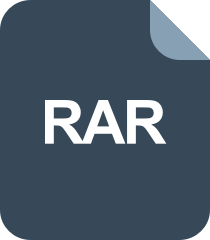
用java做的数据库的增删改查

好的,以下是一个简单的Java+sqlserver的商品信息管理系统的代码示例,它拥有增加、删除、修改和查询商品信息的功能。
首先,您需要创建一个数据库,例如,名为"product_db"的数据库,数据库中需要创建一个名为"product_table"的表,用于存储商品信息。
```
CREATE DATABASE product_db;
USE product_db;
CREATE TABLE product_table (
id INT PRIMARY KEY IDENTITY,
name VARCHAR(50),
price DECIMAL(10, 2),
description VARCHAR(255)
);
```
接下来,您可以使用Java的JDBC API连接到sqlserver数据库并执行SQL语句来实现增删改查商品信息的功能。
```
import java.sql.*;
public class ProductManager {
private static final String JDBC_DRIVER = "com.microsoft.sqlserver.jdbc.SQLServerDriver";
private static final String DB_URL = "jdbc:sqlserver://localhost:1433;databaseName=product_db";
private static final String USER = "username";
private static final String PASS = "password";
public void addProduct(String name, double price, String description) {
Connection conn = null;
PreparedStatement stmt = null;
try {
Class.forName(JDBC_DRIVER);
conn = DriverManager.getConnection(DB_URL, USER, PASS);
String sql = "INSERT INTO product_table (name, price, description) VALUES (?, ?, ?)";
stmt = conn.prepareStatement(sql);
stmt.setString(1, name);
stmt.setDouble(2, price);
stmt.setString(3, description);
stmt.executeUpdate();
} catch (SQLException se) {
se.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (stmt != null) stmt.close();
} catch (SQLException se2) {
}
try {
if (conn != null) conn.close();
} catch (SQLException se) {
se.printStackTrace();
}
}
}
public void deleteProduct(int id) {
Connection conn = null;
PreparedStatement stmt = null;
try {
Class.forName(JDBC_DRIVER);
conn = DriverManager.getConnection(DB_URL, USER, PASS);
String sql = "DELETE FROM product_table WHERE id=?";
stmt = conn.prepareStatement(sql);
stmt.setInt(1, id);
stmt.executeUpdate();
} catch (SQLException se) {
se.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (stmt != null) stmt.close();
} catch (SQLException se2) {
}
try {
if (conn != null) conn.close();
} catch (SQLException se) {
se.printStackTrace();
}
}
}
public void updateProduct(int id, String name, double price, String description) {
Connection conn = null;
PreparedStatement stmt = null;
try {
Class.forName(JDBC_DRIVER);
conn = DriverManager.getConnection(DB_URL, USER, PASS);
String sql = "UPDATE product_table SET name=?, price=?, description=? WHERE id=?";
stmt = conn.prepareStatement(sql);
stmt.setString(1, name);
stmt.setDouble(2, price);
stmt.setString(3, description);
stmt.setInt(4, id);
stmt.executeUpdate();
} catch (SQLException se) {
se.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (stmt != null) stmt.close();
} catch (SQLException se2) {
}
try {
if (conn != null) conn.close();
} catch (SQLException se) {
se.printStackTrace();
}
}
}
public void findProduct(int id) {
Connection conn = null;
PreparedStatement stmt = null;
ResultSet rs = null;
try {
Class.forName(JDBC_DRIVER);
conn = DriverManager.getConnection(DB_URL, USER, PASS);
String sql = "SELECT name, price, description FROM product_table WHERE id=?";
stmt = conn.prepareStatement(sql);
stmt.setInt(1, id);
rs = stmt.executeQuery();
while (rs.next()) {
String name = rs.getString("name");
double price = rs.getDouble("price");
String description = rs.getString("description");
System.out.printf("%s\t%.2f\t%s\n", name, price, description);
}
} catch (SQLException se) {
se.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (rs != null) rs.close();
} catch (SQLException se1) {
}
try {
if (stmt != null) stmt.close();
} catch (SQLException se2) {
}
try {
if (conn != null) conn.close();
} catch (SQLException se) {
se.printStackTrace();
}
}
}
}
```
使用上述代码,您可以创建一个`ProductManager`类,其中包含了四个方法:`addProduct`用于添加商品信息,`deleteProduct`用于删除商品信息,`updateProduct`用于更新商品信息,`findProduct`用于查询商品信息。
例如,您可以使用以下代码添加一个名为"Apple iPhone 11",价格为799.00,描述为"6.1英寸智能手机"的商品信息:
```
ProductManager manager = new ProductManager();
manager.addProduct("Apple iPhone 11", 799.00, "6.1英寸智能手机");
```
您还可以使用其他方法来删除、更新和查询商品信息。
阅读全文
相关推荐
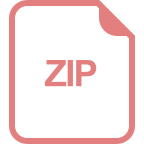
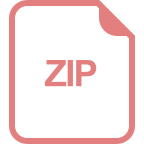
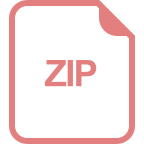
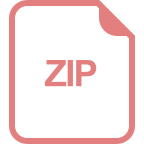
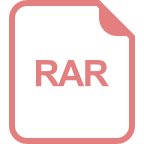
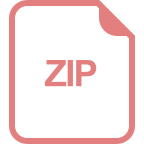
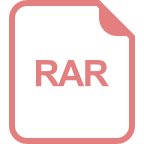
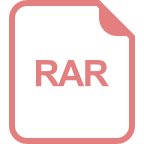
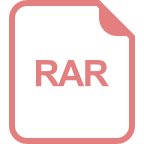
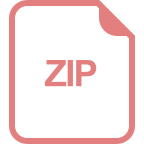
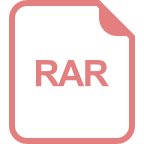
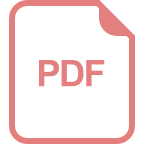
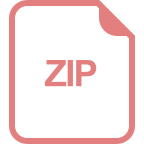
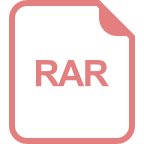
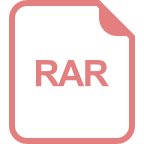
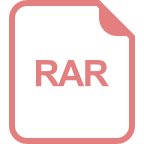