stm32printf函数的串口输出
时间: 2023-10-30 07:42:37 浏览: 148
stm32printf函数可以用来在STM32微控制器上通过串口输出数据。具体步骤如下:
1. 首先需要在代码中引入相关的库文件,如下:
#include "stdio.h"
#include "string.h"
#include "stdarg.h"
#include "stm32f10x_usart.h"
#include "stm32f10x_gpio.h"
#include "stm32f10x_rcc.h"
2. 然后需要进行串口的初始化,如下:
void USART_Config(void)
{
USART_InitTypeDef USART_InitStructure;
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_USART1, ENABLE);
// USART1_TX PA.9
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// USART1_RX PA.10
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
USART_Cmd(USART1, ENABLE);
}
3. 接下来就可以使用stm32printf函数进行输出了,如下:
void stm32printf(const char* fmt, ...)
{
char buf[256];
va_list args;
va_start(args, fmt);
vsnprintf(buf, sizeof(buf), fmt, args);
va_end(args);
int len = strlen(buf);
for (int i = 0; i < len; i++) {
while (USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET);
USART_SendData(USART1, buf[i]);
}
}
4. 最后在需要输出的地方调用stm32printf函数即可,如下:
stm32printf("Hello, world!\r\n");
注意事项:
1. 如果使用printf函数进行输出,则需要在上面的代码中添加以下语句进行重定向:
int fputc(int ch, FILE* f)
{
while (USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET);
USART_SendData(USART1, (uint8_t)ch);
return ch;
}
2. 在使用stm32printf函数进行输出时,需要注意串口的波特率和串口接口的配置要与代码中的配置相符。
阅读全文
相关推荐
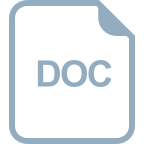
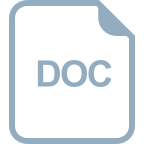
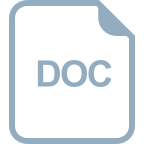
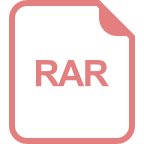
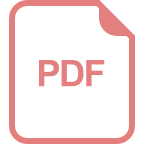
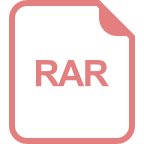
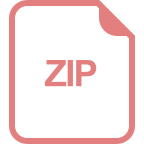


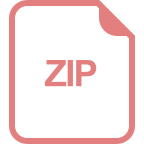
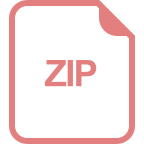





