操作系统实现如下功能:假设理发店由等待间( 2 个座位)和理发间(只有一个座位)构成,5 位顾客先后进入等待间,再进入理发间。试写出模拟理发师和顾客的程序,要求为每一个顾客创建一个线程
时间: 2024-10-24 08:17:54 浏览: 16
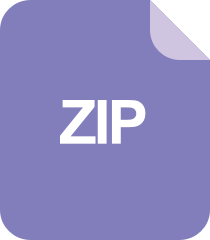
Java项目: 基于SSM框架实现的理发店会员管理系统(源码+数据库+毕业论文)

操作系统中的多线程模拟可以使用编程语言如Python来实现,这里我们采用线程池来模拟理发店的过程。下面是一个简单的Python示例:
```python
import threading
class Customer(threading.Thread):
def __init__(self, name, waiting_room, barber_shop):
super().__init__()
self.name = name
self.waiting_room = waiting_room
self.barber_shop = barber_shop
self.waiting = True
def run(self):
print(f"{self.name} 进入等待间")
self.waiting_room.append(self)
self.waiting_room.notify() # 当理发间有空闲时唤醒一个顾客
self.waiting = False
if not self.waiting_room.is_empty():
print(f"{self.name} 等待完毕,进入理发间")
self.barber_shop.take_customer(self)
else:
print(f"{self.name} 因为空等待区离开")
class WaitingRoom:
def __init__(self):
self.customers = []
self.condition = threading.Condition()
def is_empty(self):
return len(self.customers) == 0
def append(self, customer):
with self.condition:
self.customers.append(customer)
if len(self.customers) == 2:
self.condition.notify_all()
elif len(self.customers) == 1 and not self.barber_shop.customer_in_service:
self.condition.notify()
def notify(self):
with self.condition:
self.condition.notify()
class BarberShop:
def __init__(self):
self.customer_in_service = None
self.condition = threading.Condition()
def take_customer(self, customer):
with self.condition:
self.customer_in_service = customer
self.condition.notify()
while self.customer_in_service is not None:
self.condition.wait()
# 创建理发店资源
waiting_room = WaitingRoom()
barber_shop = BarberShop()
# 客户列表
customers = ["Customer1", "Customer2", "Customer3", "Customer4", "Customer5"]
# 启动客户线程
for i in customers:
Customer(i, waiting_room, barber_shop).start()
print("所有顾客服务完毕,理发店结束")
```
在这个例子中,`Customer` 类代表顾客,`WaitingRoom` 类模拟等待间,`BarberShop` 类模拟理发间。顾客会先进入等待间,当理发间有空闲时,会从等待间转移到理发间进行理发。每个顾客都是一个独立的线程。
阅读全文
相关推荐
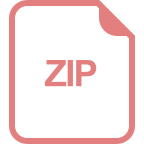
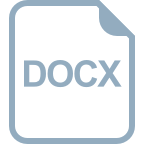


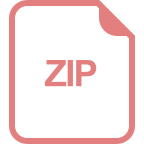
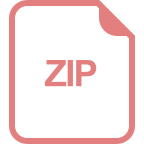
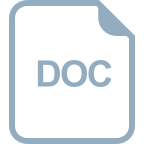
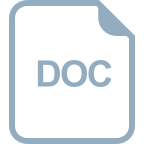
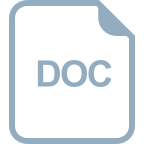
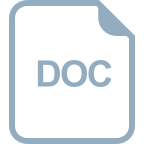







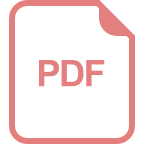