1、设计一个水果运输货车,希望货车可以运输若干种水果。要求 Java 应用程序有 5 个类,名字分别是 Fruit、Apple、Orange、 Car 和 Test,其中 Fruit 类是抽象类,Apple 类和 Orange 类继承自 Fruit 类,Car 类是描述水果运输货车,Test 是测试主类
时间: 2023-05-19 13:03:16 浏览: 67
好的,这是一个很好的编程问题。以下是一个简单的实现:
Fruit.java:
```
public abstract class Fruit {
protected String name;
protected double weight;
public Fruit(String name, double weight) {
this.name = name;
this.weight = weight;
}
public String getName() {
return name;
}
public double getWeight() {
return weight;
}
public abstract double getCost();
}
```
Apple.java:
```
public class Apple extends Fruit {
public Apple(double weight) {
super("Apple", weight);
}
public double getCost() {
return weight * 2.0; // 假设每公斤苹果的价格是 2 元
}
}
```
Orange.java:
```
public class Orange extends Fruit {
public Orange(double weight) {
super("Orange", weight);
}
public double getCost() {
return weight * 1.5; // 假设每公斤橘子的价格是 1.5 元
}
}
```
Car.java:
```
import java.util.ArrayList;
public class Car {
private ArrayList<Fruit> fruits;
public Car() {
fruits = new ArrayList<Fruit>();
}
public void addFruit(Fruit fruit) {
fruits.add(fruit);
}
public double getTotalCost() {
double totalCost = 0.0;
for (Fruit fruit : fruits) {
totalCost += fruit.getCost();
}
return totalCost;
}
}
```
Test.java:
```
public class Test {
public static void main(String[] args) {
Car car = new Car();
car.addFruit(new Apple(2.5));
car.addFruit(new Orange(3.0));
System.out.println("Total cost: " + car.getTotalCost());
}
}
```
这个程序可以创建一个水果运输货车,可以添加苹果和橘子,计算总成本。
相关推荐
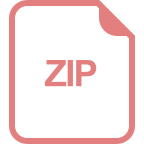














