c# 中文填空题编程 每条中文填空题有1个要填中文的空 编程 程序 先由管理员出10题 放入题库文件中 用户登录随机抽5题 输入中文答案 每个空对加3分 统计用户答对题目题次数 统计得分 含代码注释
时间: 2024-02-13 22:02:59 浏览: 23
好的,让我来为您解答。以下是一个示例程序,可供参考:
```csharp
using System;
using System.Collections.Generic;
using System.IO;
namespace ChineseFillInBlankQuiz
{
class Program
{
static void Main(string[] args)
{
// 出题
List<Question> questions = GenerateQuestions(10);
// 将题目保存到文件中
SaveQuestions(questions, "questions.txt");
// 登录
Console.Write("请输入用户名:");
string username = Console.ReadLine();
// 抽题
List<Question> selectedQuestions = SelectQuestions("questions.txt", 5);
// 答题
int score = 0;
foreach (Question question in selectedQuestions)
{
Console.WriteLine(question.Content);
Console.Write("请填写空缺处的中文:");
string answer = Console.ReadLine();
if (answer == question.Answer)
{
Console.WriteLine("回答正确!");
score += 3;
question.CorrectCount++;
}
else
{
Console.WriteLine("回答错误。正确答案是:" + question.Answer);
}
question.TotalCount++;
}
// 输出成绩和统计信息
Console.WriteLine("您的得分是:" + score);
Console.WriteLine("您的答题情况如下:");
foreach (Question question in selectedQuestions)
{
Console.WriteLine(question.Content + ",回答次数:" + question.TotalCount + ",回答正确次数:" + question.CorrectCount);
}
Console.ReadLine();
}
// 生成题目
static List<Question> GenerateQuestions(int count)
{
List<Question> questions = new List<Question>();
for (int i = 1; i <= count; i++)
{
Question question = new Question();
question.Content = "第" + i + "题:请填写空缺处的中文。";
question.Answer = "答案" + i;
questions.Add(question);
}
return questions;
}
// 将题目保存到文件中
static void SaveQuestions(List<Question> questions, string filename)
{
using (StreamWriter writer = new StreamWriter(filename))
{
foreach (Question question in questions)
{
writer.WriteLine(question.Content);
writer.WriteLine(question.Answer);
}
}
}
// 从文件中随机抽题
static List<Question> SelectQuestions(string filename, int count)
{
List<Question> questions = new List<Question>();
using (StreamReader reader = new StreamReader(filename))
{
string content = null;
string answer = null;
while ((content = reader.ReadLine()) != null && (answer = reader.ReadLine()) != null)
{
Question question = new Question();
question.Content = content;
question.Answer = answer;
questions.Add(question);
}
}
List<Question> selectedQuestions = new List<Question>();
Random random = new Random();
for (int i = 0; i < count; i++)
{
int index = random.Next(questions.Count);
selectedQuestions.Add(questions[index]);
questions.RemoveAt(index);
}
return selectedQuestions;
}
}
// 题目类
class Question
{
public string Content { get; set; }
public string Answer { get; set; }
public int TotalCount { get; set; }
public int CorrectCount { get; set; }
}
}
```
程序的核心是 `GenerateQuestions`、`SaveQuestions` 和 `SelectQuestions` 三个函数,分别用于生成题目、将题目保存到文件中和从文件中随机抽题。`Question` 类用于存储题目的内容、答案和统计信息。在 `Main` 函数中,先生成10道题目,然后将题目保存到文件 `questions.txt` 中。用户登录后,程序从文件中随机抽取5道题目进行答题。每回答一道题目,程序会判断答案是否正确,然后更新题目的统计信息。最后输出得分和答题情况。
请注意,程序中的题目和答案都是示例数据,实际应用中需要根据具体需求进行修改。此外,程序还可以加入其他功能,如让管理员从文件中读取题目,并可以修改已有的题目或添加新的题目。
相关推荐
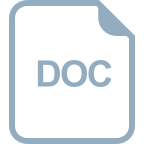
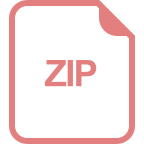
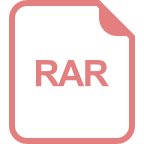
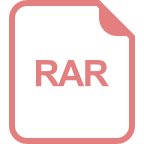
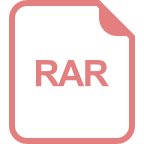
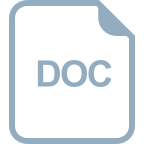
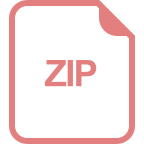
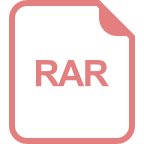
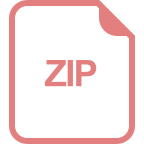
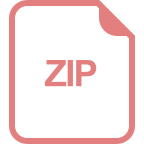