请编写一个工具类,实现spring程序的注解扫描和自动装配
时间: 2024-02-25 07:55:50 浏览: 30
好的,以下是一个简单的工具类实现:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.stereotype.Component;
import java.lang.reflect.Field;
@ComponentScan("com.example") // 指定扫描的包路径
public class SpringUtils {
private static ApplicationContext applicationContext;
static {
applicationContext = new AnnotationConfigApplicationContext(SpringUtils.class);
}
public static <T> T getBean(Class<T> clazz) {
return applicationContext.getBean(clazz);
}
public static void autowire(Object object) throws IllegalAccessException {
Class<?> clazz = object.getClass();
Field[] fields = clazz.getDeclaredFields();
for (Field field : fields) {
if (field.isAnnotationPresent(Autowired.class)) {
Object bean = getBean(field.getType());
field.setAccessible(true);
field.set(object, bean);
}
}
}
}
```
使用方法:
1. 在需要进行注解扫描和自动装配的包路径上添加`@ComponentScan("com.example")`注解。
2. 在需要自动装配的类中使用`@Autowired`注解标记需要自动装配的属性。
3. 在需要使用自动装配的类中,调用`SpringUtils.autowire(object)`方法进行自动装配。
4. 在需要获取`Bean`的类中,调用`SpringUtils.getBean(clazz)`方法获取`Bean`对象。
注意事项:
1. 需要在Spring容器启动之后才能进行自动装配,否则会抛出异常。
2. 需要保证需要自动装配的类都是Spring容器中的`Bean`,否则会抛出异常。
3. 需要保证需要自动装配的属性是Spring容器中的`Bean`,否则会抛出异常。
4. 需要保证需要获取的`Bean`对象在Spring容器中存在,否则会抛出异常。
相关推荐
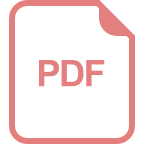
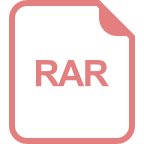














