springboot 整合 redis, 如何实现redis批量删除working开头的key
时间: 2024-09-25 09:14:48 浏览: 30
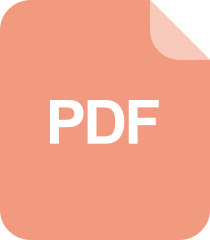
微服务SpringBoot整合Redis基于Redis的Stream消息队列实现异步秒杀下单

Spring Boot整合Redis时,为了实现批量删除以"working-"开头的键,你可以按照以下步骤操作:
1. **添加依赖**:
首先,在你的`pom.xml`文件中添加Spring Data Redis和Jedis库的依赖,如果还没有,加入如下内容:
```xml
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-redis</artifactId>
</dependency>
<!-- 如果需要使用Jedis客户端 -->
<dependency>
<groupId>redis.clients</groupId>
<artifactId>jedis</artifactId>
</dependency>
</dependencies>
```
2. **配置Redis连接**:
在`application.properties`或`application.yml`中配置Redis的连接信息,例如:
```properties
spring.redis.host=localhost
spring.redis.port=6379
```
3. **编写服务类**:
创建一个服务类,利用@Autowired注入RedisTemplate或者JedisClient,然后定义一个方法来删除键:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.stereotype.Service;
@Service
public class RedisKeyDeleter {
@Autowired
private RedisTemplate<String, String> redisTemplate; // 或者 JedisClient jedis;
public void deleteWorkingKeys() {
Set<String> keysToDelete = redisTemplate.keys("working:*");
if (!keysToDelete.isEmpty()) {
redisTemplate.delete(keysToDelete);
System.out.println("Deleted working keys: " + keysToDelete);
} else {
System.out.println("No 'working-' keys found to delete.");
}
}
}
```
4. **调用方法**:
在需要删除键的地方,如控制器或其他业务处理类中,通过`@Autowired`注入该服务,并调用`deleteWorkingKeys()`方法。
5. **异常处理**:
可能会遇到连接异常或权限等问题,记得添加适当的错误处理和日志记录。
阅读全文
相关推荐
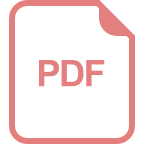
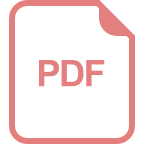
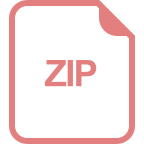
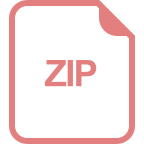
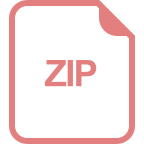
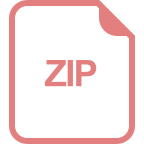
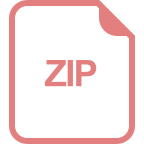
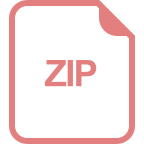