C# opencv rstp取视频流并显示在pictureBox上
时间: 2024-04-15 07:26:56 浏览: 296
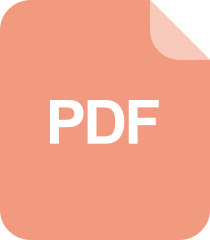
opencv 获取rtsp流媒体视频的实现方法

要在C#中使用OpenCV库获取RTSP视频流并将其显示在PictureBox上,你需要使用OpenCV的VideoCapture类来打开RTSP流,并使用OpenCV的Mat类来处理视频帧,并将其转换为图像以在PictureBox上显示。以下是一个示例代码:
首先,确保你已经安装了OpenCV并将其配置到你的C#项目中。你可以使用NuGet包管理器来安装OpenCvSharp库。
然后,在Windows Forms应用程序中,你需要添加一个PictureBox控件和一个Button控件。当点击按钮时,将触发获取RTSP视频流并显示在PictureBox上的操作。
```csharp
using System;
using System.Drawing;
using System.Windows.Forms;
using OpenCvSharp;
namespace RTSPPlayer
{
public partial class Form1 : Form
{
private VideoCapture videoCapture;
private bool isPlaying;
public Form1()
{
InitializeComponent();
}
private void btnStart_Click(object sender, EventArgs e)
{
string rtspUrl = "rtsp://example.com/stream"; // RTSP流的URL
videoCapture = new VideoCapture(rtspUrl);
isPlaying = true;
// 创建一个定时器,在固定的时间间隔内刷新视频帧
Timer timer = new Timer();
timer.Interval = 33; // 每秒约30帧
timer.Tick += Timer_Tick;
timer.Start();
}
private void Timer_Tick(object sender, EventArgs e)
{
if (isPlaying)
{
// 从视频捕捉设备中获取下一帧
Mat frame = new Mat();
videoCapture.Read(frame);
// 将帧转换为图像
Bitmap image = OpenCvSharp.Extensions.BitmapConverter.ToBitmap(frame);
// 在PictureBox上显示图像
pictureBox.Image = image;
// 释放帧资源
frame.Release();
}
}
private void btnStop_Click(object sender, EventArgs e)
{
isPlaying = false;
// 释放视频捕捉设备资源
if (videoCapture != null)
{
videoCapture.Release();
videoCapture.Dispose();
videoCapture = null;
}
// 清空PictureBox的图像
pictureBox.Image = null;
}
}
}
```
在上述代码中,我们使用OpenCvSharp库创建一个VideoCapture对象来打开RTSP流。然后,我们使用一个定时器来从视频捕捉设备中获取下一帧,并将其转换为图像以在PictureBox上显示。
请注意,上述代码只是一个简单的示例,你可能需要根据实际需求进行修改和扩展。另外,为了避免阻塞UI线程,你可能需要使用异步操作来获取视频帧数据。
希望这可以帮助到你!
阅读全文
相关推荐
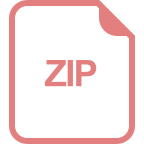
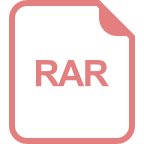
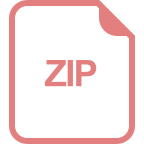
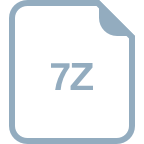
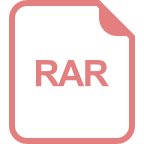
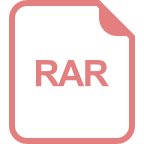
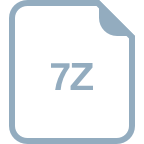
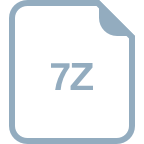
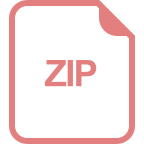
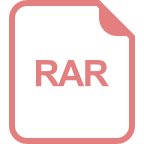
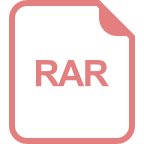
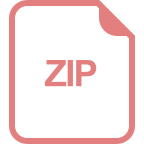
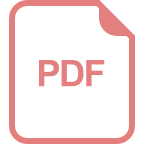
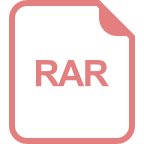
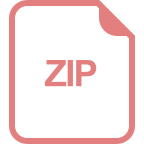