HttpsURLConnection 跳过证书校验
时间: 2023-08-22 10:09:06 浏览: 98
在Java中,HttpsURLConnection默认会验证服务器的证书,防止中间人攻击。如果你的HTTPS服务器使用的是自签名证书或者其他不受信任的证书,那么HttpsURLConnection会抛出javax.net.ssl.SSLHandshakeException异常。
如果你想跳过证书校验,可以使用以下代码:
```java
import java.net.URL;
import javax.net.ssl.HttpsURLConnection;
import javax.net.ssl.SSLContext;
import javax.net.ssl.TrustManager;
import javax.net.ssl.X509TrustManager;
import java.security.cert.X509Certificate;
public class HttpsURLConnectionExample {
public static void main(String[] args) throws Exception {
String url = "https://www.example.com/api";
URL obj = new URL(url);
// 创建一个信任管理器,跳过证书校验
TrustManager[] trustAllCerts = new TrustManager[] {
new X509TrustManager() {
public void checkClientTrusted(X509Certificate[] chain, String authType) {}
public void checkServerTrusted(X509Certificate[] chain, String authType) {}
public X509Certificate[] getAcceptedIssuers() { return new X509Certificate[0]; }
}
};
// 创建一个SSL上下文对象,跳过证书校验
SSLContext sslContext = SSLContext.getInstance("SSL");
sslContext.init(null, trustAllCerts, new java.security.SecureRandom());
// 创建一个HttpsURLConnection对象,使用上面创建的SSL上下文对象
HttpsURLConnection.setDefaultSSLSocketFactory(sslContext.getSocketFactory());
HttpsURLConnection con = (HttpsURLConnection) obj.openConnection();
// 设置请求方法
con.setRequestMethod("GET");
// 打印响应状态码
int responseCode = con.getResponseCode();
System.out.println("Response Code : " + responseCode);
// 读取响应内容
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
// 打印响应内容
System.out.println(response.toString());
}
}
```
在上面的示例代码中,我们创建了一个信任管理器,它跳过了证书校验。然后我们创建了一个SSL上下文对象,并使用上面创建的信任管理器初始化它。最后,我们将这个SSL上下文对象设置为默认的SSL套接字工厂,并使用它来创建一个HttpsURLConnection对象。这样我们就可以跳过证书校验,并正常使用HTTPS接口了。
相关推荐
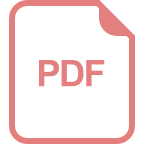
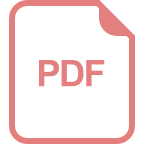
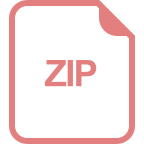














