高精度运算C++代码
时间: 2023-07-10 21:22:18 浏览: 70
以下是一个简单的高精度加法示例代码,你可以根据需要进行修改和扩展:
```c++
#include <iostream>
#include <cstring>
using namespace std;
const int MAXN = 1005;
const int BASE = 10;
struct BigInt {
int len, s[MAXN];
BigInt() {
memset(s, 0, sizeof(s));
len = 1;
}
BigInt(int num) {
*this = num;
}
BigInt(const char* num) {
*this = num;
}
BigInt operator = (int num) {
char s[MAXN];
sprintf(s, "%d", num);
*this = s;
return *this;
}
BigInt operator = (const char* num) {
memset(s, 0, sizeof(s));
len = strlen(num);
for (int i = 0; i < len; i++) s[i] = num[len - i - 1] - '0';
return *this;
}
BigInt operator + (const BigInt& b) const {
BigInt c;
c.len = 0;
for (int i = 0, g = 0; g || i < max(len, b.len); i++) {
int x = g;
if (i < len) x += s[i];
if (i < b.len) x += b.s[i];
c.s[c.len++] = x % BASE;
g = x / BASE;
}
return c;
}
void clean() {
while (len > 1 && !s[len-1]) len--;
}
BigInt operator * (const BigInt& b) const {
BigInt c; c.len = len + b.len;
for (int i = 0; i < len; i++)
for (int j = 0; j < b.len; j++)
c.s[i+j] += s[i] * b.s[j];
for (int i = 0; i < c.len-1; i++)
c.s[i+1] += c.s[i] / BASE, c.s[i] %= BASE;
c.clean();
return c;
}
BigInt operator - (const BigInt& b) const {
BigInt c; c.len = 0;
for (int i = 0, g = 0; i < len; i++) {
int x = s[i] - g;
if (i < b.len) x -= b.s[i];
if (x >= 0) g = 0;
else g = 1, x += BASE;
c.s[c.len++] = x;
}
c.clean();
return c;
}
bool operator < (const BigInt& b) const {
if (len != b.len) return len < b.len;
for (int i = len-1; i >= 0; i--)
if (s[i] != b.s[i]) return s[i] < b.s[i];
return false;
}
bool operator > (const BigInt& b) const {
return b < *this;
}
bool operator <= (const BigInt& b) const {
return !(b < *this);
}
bool operator == (const BigInt& b) const {
return !(b < *this) && !(*this < b);
}
BigInt operator += (const BigInt& b) {
*this = *this + b;
return *this;
}
friend istream& operator >> (istream& in, BigInt& x) {
string s;
in >> s;
x = s.c_str();
return in;
}
friend ostream& operator << (ostream& out, const BigInt& x) {
out << x.s[x.len-1];
for (int i = x.len-2; i >= 0; i--)
out << x.s[i];
return out;
}
};
int main() {
BigInt a, b;
cin >> a >> b;
cout << a + b << endl;
return 0;
}
```
注意:此代码仅作为参考,仍需根据实际情况或需求进行修改和优化。
相关推荐
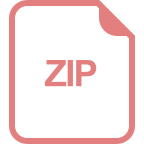













