多个Servlet通过ServletContext对象实现数据共享
时间: 2024-01-01 20:19:57 浏览: 41
多个Servlet可以通过ServletContext对象实现数据共享。ServletContext对象代表了整个Web应用程序的上下文环境,可以在多个Servlet之间共享数据。
通过ServletContext对象,可以使用以下方法实现数据共享:
1. setAttribute(String name, Object value):将一个属性值存储在ServletContext对象中。可以在不同的Servlet中使用相同的名称来访问这个属性值。
2. getAttribute(String name):根据指定的属性名称从ServletContext对象中获取属性值。
3. removeAttribute(String name):根据指定的属性名称从ServletContext对象中移除属性值。
实现数据共享的步骤如下:
1. 在一个Servlet中设置属性值,使用setAttrbute()方法将属性存储在ServletContext对象中。
2. 在另一个Servlet中获取属性值,使用getAttribute()方法从ServletContext对象中获取属性值。
3. 可以在需要的时候修改或移除属性值,使用setAttribute()或removeAttribute()方法进行操作。
相关问题
用Java举出代码例子,在多个个servlet中怎么用application共享同一个数据
以下是一个 Java 代码例子,演示如何在多个 servlet 中使用 application 共享同一个数据:
```
public class MyServletContextListener implements ServletContextListener {
public void contextInitialized(ServletContextEvent sce) {
// 在 ServletContext 中存储需要共享的数据
ServletContext context = sce.getServletContext();
context.setAttribute("counter", 0);
}
public void contextDestroyed(ServletContextEvent sce) {
// Do nothing
}
}
```
在这个例子中,我们定义了一个 ServletContextListener,当 web 应用程序启动时,我们在 ServletContext 中存储一个名为 "counter" 的变量,并初始化为 0。当 web 应用程序关闭时,我们不需要做任何操作。
接下来,我们在多个 servlet 中使用这个变量:
```
public class FirstServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// 获取 ServletContext 对象
ServletContext context = getServletContext();
// 获取计数器的值并自增
int counter = (int) context.getAttribute("counter");
counter++;
context.setAttribute("counter", counter);
// 输出计数器的值到响应中
response.setContentType("text/plain");
PrintWriter out = response.getWriter();
out.println("Counter: " + counter);
}
}
public class SecondServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// 获取 ServletContext 对象
ServletContext context = getServletContext();
// 获取计数器的值
int counter = (int) context.getAttribute("counter");
// 输出计数器的值到响应中
response.setContentType("text/plain");
PrintWriter out = response.getWriter();
out.println("Counter: " + counter);
}
}
```
在这个例子中,当用户访问 FirstServlet 时,我们从 ServletContext 中获取计数器的值,并自增,然后再存储回 ServletContext 中。当用户访问 SecondServlet 时,我们从 ServletContext 中获取计数器的值,并输出到响应中。这样,我们就可以在多个 servlet 中共享同一个数据了。
需要注意的是,在整个 web 应用程序中,ServletContext 对象是唯一的,因此存储在其中的数据在整个应用程序中都是可见的。为了避免数据冲突和安全问题,我们需要小心地使用 ServletContext 中的数据。
servlet的内置对象
在Servlet中,有以下9个内置对象:
1. request:表示客户端的请求,可以获取请求参数、请求头、请求方法等信息。
2. response:表示服务器的响应,可以设置响应头、响应状态码、发送响应数据等。
3. session:表示会话,可以在多个请求之间共享数据。
4. application:表示应用程序,可以在整个应用程序中共享数据。
5. out:表示输出流,可以向客户端发送响应数据。
6. config:表示Servlet的配置信息,可以获取Servlet的初始化参数等。
7. servletContext:表示Servlet上下文,可以在整个应用程序中共享数据。
8. pageContext:表示页面上下文,可以获取当前页面的信息,如请求参数、请求头等。
9. exception:表示异常对象,可以获取异常堆栈信息等。
这些内置对象在Servlet开发中非常重要,可以帮助我们快速、方便地处理各种请求,并向客户端发送响应数据。
相关推荐
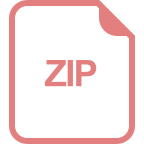












