c++ 非线性函数拟合 代码
时间: 2023-08-30 13:12:43 浏览: 83
当涉及到非线性函数拟合时,常用的方法是最小二乘法。在C++中,可以使用优化库(如Eigen或Ceres Solver)来实现这个任务。下面是一个使用Eigen库进行非线性函数拟合的示例代码:
```cpp
#include <iostream>
#include <Eigen/Dense>
#include <unsupported/Eigen/NonLinearOptimization>
using namespace std;
using namespace Eigen;
typedef Eigen::VectorXd Vector;
// 定义非线性函数模型
struct MyFunctor
{
int operator()(const Vector &x, Vector &fvec) const
{
double a = x(0);
double b = x(1);
// 非线性方程模型
fvec(0) = exp(a * b) - 1.0;
fvec(1) = a * a + b * b - 3.0;
return 0;
}
};
int main()
{
Vector x(2); // 待拟合的参数
x << 1.0, 1.0;
MyFunctor functor; // 定义函数模型对象
LevenbergMarquardt<MyFunctor> lm(functor); // 使用Levenberg-Marquardt算法进行拟合
lm.parameters.maxfev = 1000; // 设置最大迭代次数
lm.parameters.xtol = 1.0e-10; // 设置迭代停止条件
int info = lm.minimize(x); // 执行拟合
if (info == LevenbergMarquardtSpace::Status::ImproperInputParameters)
{
cout << "Invalid input parameters" << endl;
}
else if (info == LevenbergMarquardtSpace::Status::TooManyFunctionEvaluation)
{
cout << "Too many function evaluations" << endl;
}
else if (info == LevenbergMarquardtSpace::Status::ImproperInputParameters)
{
cout << "Input variables contain NaN or Inf" << endl;
}
else if (info == LevenbergMarquardtSpace::Status::RelativeReductionTooSmall)
{
cout << "Relative reduction too small" << endl;
}
else if (info == LevenbergMarquardtSpace::Status::RelativeErrorTooSmall)
{
cout << "Relative error too small" << endl;
}
else if (info == LevenbergMarquardtSpace::Status::CosinusTooSmall)
{
cout << "Cosinus too small" << endl;
}
else if (info == LevenbergMarquardtSpace::Status::Success)
{
cout << "Optimization success!" << endl;
cout << "a = " << x(0) << endl;
cout << "b = " << x(1) << endl;
}
else
{
cout << "Unknown error" << endl;
}
return 0;
}
```
这个示例代码中,我们定义了一个包含两个未知参数的非线性方程模型。然后使用Levenberg-Marquardt算法进行拟合,得到参数的估计值。你可以根据自己的需求修改和扩展这段代码,以适应不同的非线性函数拟合任务。
相关推荐
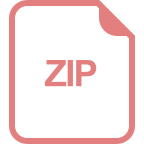
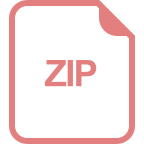
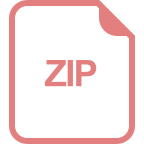
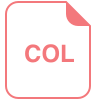
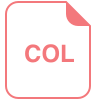












