Android studio 按钮实现照片切换
时间: 2023-12-20 15:04:05 浏览: 86
要实现照片切换,你可以使用一个 ImageView 来显示当前的照片,并在按钮的点击事件中更改 ImageView 的内容。以下是一个简单的实现步骤:
1. 在你的布局文件中添加一个 ImageView 元素,用于显示照片:
```xml
<ImageView
android:id="@+id/imageView"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:src="@drawable/photo1" />
```
2. 在你的 Activity 中获取 ImageView 的实例,并创建一个数组来存储所有的照片资源 ID:
```java
private ImageView imageView;
private int[] photoIds = { R.drawable.photo1, R.drawable.photo2, R.drawable.photo3 };
private int currentPhotoIndex = 0;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
imageView = findViewById(R.id.imageView);
}
```
3. 在你的按钮的点击事件中更改 ImageView 的内容:
```java
public void onNextButtonClick(View view) {
// 切换到下一张照片
currentPhotoIndex++;
if (currentPhotoIndex >= photoIds.length) {
currentPhotoIndex = 0; // 循环切换照片
}
imageView.setImageResource(photoIds[currentPhotoIndex]);
}
public void onPrevButtonClick(View view) {
// 切换到上一张照片
currentPhotoIndex--;
if (currentPhotoIndex < 0) {
currentPhotoIndex = photoIds.length - 1; // 循环切换照片
}
imageView.setImageResource(photoIds[currentPhotoIndex]);
}
```
在这个例子中,我们创建了一个包含三张照片的数组,然后通过两个按钮实现了向前和向后切换照片的功能。你可以根据你的实际需求来调整这些代码。
阅读全文
相关推荐

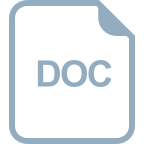













