写一个深度神经网络的图像匹配算法
时间: 2023-11-18 18:23:22 浏览: 36
图像匹配是计算机视觉领域中的一项重要任务,通过将两幅图像进行比较,找到它们之间的相似度或匹配程度。深度神经网络已经在图像匹配任务中取得了很好的效果,下面是一个基于深度神经网络的图像匹配算法的基本步骤:
1. 数据准备:准备两幅图像作为输入数据,对这两幅图像进行预处理,包括图像缩放、裁剪等操作。
2. 特征提取:使用深度卷积神经网络(CNN)对两幅图像进行特征提取。对于每幅图像,将其输入到CNN中,得到一个固定维度的特征向量。可以使用预训练好的CNN模型,例如VGG、ResNet等。
3. 特征匹配:对两幅图像的特征向量进行匹配。常用的方法包括余弦相似度、欧式距离等。
4. 匹配结果输出:根据特征匹配的结果,输出两幅图像之间的相似度或匹配程度。
下面是一个简单的Python代码示例,演示了如何使用预训练好的VGG16模型进行图像匹配:
```python
import numpy as np
import tensorflow as tf
from tensorflow.keras.applications.vgg16 import preprocess_input, VGG16
# Load VGG16 model
model = VGG16(weights='imagenet', include_top=False)
# Load two images and preprocess them
img1 = tf.keras.preprocessing.image.load_img('image1.jpg', target_size=(224, 224))
img1 = tf.keras.preprocessing.image.img_to_array(img1)
img1 = np.expand_dims(img1, axis=0)
img1 = preprocess_input(img1)
img2 = tf.keras.preprocessing.image.load_img('image2.jpg', target_size=(224, 224))
img2 = tf.keras.preprocessing.image.img_to_array(img2)
img2 = np.expand_dims(img2, axis=0)
img2 = preprocess_input(img2)
# Extract features for image 1 and image 2
feat1 = model.predict(img1)
feat2 = model.predict(img2)
# Compute cosine similarity between features
similarity = np.dot(feat1, feat2.T) / (np.linalg.norm(feat1) * np.linalg.norm(feat2))
print('Similarity between image 1 and image 2:', similarity)
```
在上面的代码中,我们使用了VGG16模型对两幅图像进行特征提取,并计算了它们之间的余弦相似度作为相似度的度量。实际上,在实际应用中,可能需要根据具体的场景和需求,使用不同的深度网络模型和特征匹配方法来实现图像匹配。
相关推荐
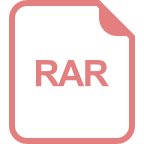
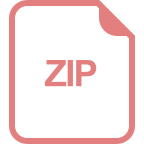
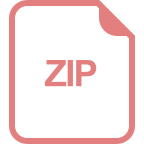














