单字母替换加密方法——单表置换密码C#
时间: 2023-09-11 14:04:20 浏览: 58
单字母替换加密方法又称为单表置换密码,是一种简单的加密方法。它的加密过程是将明文中的每个字母按照一定规律替换成密文中的另一个字母。
下面是一个使用C#实现的单字母替换加密方法:
```csharp
using System;
class Program
{
static void Main(string[] args)
{
string plaintext = "HELLO WORLD"; // 明文
string key = "QWERTYUIOPASDFGHJKLZXCVBNM"; // 密钥
string ciphertext = Encrypt(plaintext, key); // 加密
Console.WriteLine("密文:" + ciphertext);
string decrypttext = Decrypt(ciphertext, key); // 解密
Console.WriteLine("解密后明文:" + decrypttext);
Console.ReadKey();
}
// 加密函数
static string Encrypt(string plaintext, string key)
{
string ciphertext = "";
plaintext = plaintext.ToUpper(); // 将明文转换成大写
for (int i = 0; i < plaintext.Length; i++)
{
char c = plaintext[i];
if (c >= 'A' && c <= 'Z')
{
int index = c - 'A'; // 明文字母在字母表中的位置
ciphertext += key[index];
}
else
{
ciphertext += c;
}
}
return ciphertext;
}
// 解密函数
static string Decrypt(string ciphertext, string key)
{
string plaintext = "";
ciphertext = ciphertext.ToUpper(); // 将密文转换成大写
for (int i = 0; i < ciphertext.Length; i++)
{
char c = ciphertext[i];
if (c >= 'A' && c <= 'Z')
{
int index = key.IndexOf(c); // 密文字母在密钥中的位置
plaintext += (char)('A' + index);
}
else
{
plaintext += c;
}
}
return plaintext;
}
}
```
这个程序使用了一个字母表(即26个大写字母)作为明文和密文的取值范围,使用一个密钥来表示替换规律。在加密时,对于明文中的每个字母,如果是字母表中的字母,则在密钥中找到相应位置上的字母作为密文对应的字母;否则直接将该字符作为密文对应的字符。在解密时,对于密文中的每个字母,如果是密钥中的字母,则在字母表中找到相应位置上的字母作为明文对应的字母;否则直接将该字符作为明文对应的字符。
需要注意的是,密钥中的每个字母都只能出现一次,否则解密时会出现歧义。
相关推荐
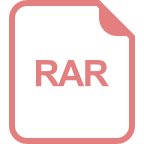













