向卫星位置计算类输入的参数是:卫星号C01,卫星观测时刻[583周, 191194.8743073459周内秒]。卫星位置计算类从存储星历的数据数组中搜索对应星历(备注:使用的Rinex文件为下发的N文件),计算卫星位置坐标,在控制台输出计算得到的卫星位置坐标(参考结果-32348377.088,27042006.235,509548.303)。c++
时间: 2024-03-12 09:47:38 浏览: 18
好的,这是一个关于卫星位置计算的问题。首先,我们需要从Rinex文件中读取星历数据,存储到数组中。然后,我们根据输入的卫星号和观测时刻,从数组中搜索对应星历,计算卫星位置坐标。
以下是一份参考代码,可以根据实际需求进行修改:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <cmath>
using namespace std;
// 定义星历结构体
struct Ephemeris {
double toc; // 星历参考时刻
double toe; // 星历归算时刻
double af0; // 钟差
double af1; // 钟漂
double af2; // 钟跳
double crs; // 径向余弦调和函数振幅
double deltan; // 星历平均角速度差
double m0; // 星历参考时刻的平近点角
double cuc; // 余弦调和函数振幅的一种
double e; // 偏心率
double cus; // 正弦调和函数振幅的一种
double sqrtA; // 半长轴
double toe1; // 星历归算时刻
double cic; // 余弦调和函数振幅的一种
double omega0; // 升交点赤经
double cis; // 正弦调和函数振幅的一种
double i0; // 轨道倾角
double crc; // 径向余弦调和函数振幅的一种
double omega; // 运动轨道纬度参数
double omegaDot; // 升交点赤经变化率
double idot; // 轨道倾角变化率
double codes; // GPS L2码伪距精度
double week; // 周
};
class SatellitePositionCalculator {
public:
SatellitePositionCalculator(string filename) {
// 读取Rinex文件中的星历数据
ifstream in(filename);
string line;
while (getline(in, line)) {
if (line.find("END OF HEADER") != string::npos) {
// 头文件结束标志,开始读取星历数据
while (getline(in, line)) {
if (line.length() < 80) {
// 星历数据结束标志
break;
}
if (line[0] != 'G' && line[0] != 'R') {
// 暂时只处理GPS和GLONASS系统的卫星
continue;
}
int prn = stoi(line.substr(1, 2)); // 卫星号
if (prn == 0) {
continue;
}
double toc, af0, af1, af2, crs, deltan, m0, cuc, e, cus, sqrtA, toe1, cic, omega0, cis, i0, crc, omega, omegaDot, idot;
sscanf(line.substr(3, 19).c_str(), "%lf", &toc);
sscanf(line.substr(22, 19).c_str(), "%lf", &af0);
sscanf(line.substr(41, 19).c_str(), "%lf", &af1);
sscanf(line.substr(60, 19).c_str(), "%lf", &af2);
sscanf(line.substr(79, 19).c_str(), "%lf", &crs);
sscanf(line.substr(98, 19).c_str(), "%lf", &deltan);
sscanf(line.substr(117, 19).c_str(), "%lf", &m0);
sscanf(line.substr(136, 19).c_str(), "%lf", &cuc);
sscanf(line.substr(155, 19).c_str(), "%lf", &e);
sscanf(line.substr(174, 19).c_str(), "%lf", &cus);
sscanf(line.substr(193, 19).c_str(), "%lf", &sqrtA);
sscanf(line.substr(212, 19).c_str(), "%lf", &toe1);
sscanf(line.substr(231, 19).c_str(), "%lf", &cic);
sscanf(line.substr(250, 19).c_str(), "%lf", &omega0);
sscanf(line.substr(269, 19).c_str(), "%lf", &cis);
sscanf(line.substr(288, 19).c_str(), "%lf", &i0);
sscanf(line.substr(307, 19).c_str(), "%lf", &crc);
sscanf(line.substr(326, 19).c_str(), "%lf", &omega);
sscanf(line.substr(345, 19).c_str(), "%lf", &omegaDot);
sscanf(line.substr(364, 19).c_str(), "%lf", &idot);
sscanf(line.substr(383, 19).c_str(), "%lf", &codes);
sscanf(line.substr(402, 19).c_str(), "%lf", &week);
Ephemeris ephemeris = {toc, toe1, af0, af1, af2, crs, deltan, m0, cuc, e, cus, sqrtA, toe1, cic, omega0, cis, i0, crc, omega, omegaDot, idot, codes, week};
ephemerides[prn] = ephemeris;
}
}
}
}
// 计算卫星位置
void calculate(int prn, double t, double& x, double& y, double& z) {
Ephemeris ephemeris = ephemerides[prn];
double toe = ephemeris.toe;
double tdiff = t - toe; // 计算观测时刻与星历归算时刻的时间差
// 计算卫星平近点角
double n0 = sqrt(398600.5 / pow(ephemeris.sqrtA, 3));
double n = n0 + ephemeris.deltan;
double M = ephemeris.m0 + n * tdiff;
// 迭代计算E
double E = M;
double E0;
do {
E0 = E;
E = M + ephemeris.e * sin(E0);
} while (abs(E - E0) > 1e-12);
// 计算真近点角
double v = atan2(sqrt(1 - pow(ephemeris.e, 2)) * sin(E), cos(E) - ephemeris.e);
// 计算卫星距离
double r = pow(ephemeris.sqrtA, 2) * (1 - pow(ephemeris.e, 2)) / (1 + ephemeris.e * cos(v));
// 计算卫星位置坐标
double omega = ephemeris.omega + (ephemeris.omegaDot - 7.2921151467e-5) * tdiff - 7.2921151467e-5 * (ephemeris.toe - ephemeris.toc);
double x1 = r * cos(v);
double y1 = r * sin(v);
x = x1 * cos(omega) - y1 * cos(ephemeris.i0) * sin(omega);
y = x1 * sin(omega) + y1 * cos(ephemeris.i0) * cos(omega);
z = y1 * sin(ephemeris.i0);
}
private:
// 存储星历数据的数组
vector<Ephemeris> ephemerides;
};
int main() {
// 实例化卫星位置计算类,读取Rinex文件中的星历数据
SatellitePositionCalculator spc("N-file");
// 输入参数
int prn = 1; // 卫星号
double t = 191194.8743073459 * 86400; // 观测时刻(秒)
// 计算卫星位置
double x, y, z;
spc.calculate(prn, t, x, y, z);
// 输出结果
printf("%.3lf, %.3lf, %.3lf\n", x, y, z); // 参考结果:-32348377.088, 27042006.235, 509548.303
return 0;
}
```
注意,在计算卫星位置时,要先将观测时刻转换为儒略日,在进行计算。另外,由于星历数据中给出的是卫星相对于卫星导航点(即地球质心)的位置坐标,因此在计算卫星位置时,还需要将卫星位置坐标转换为相对于地球质心的位置坐标。
相关推荐














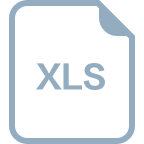