#include<iostream> using namespace std; #include<iostream> using namespace std; //在此处添加代码 class Line: public Shape{ public: void Show(){cout<<"Line"<<endl;} }; class Rect: public Shape{ public: void show(){cout<<"Rect"<<endl;} }; int main(){ Shape *p = new Line(); p->Show(); delete p; Shape *p = new Rect(); p->Show(); delete p; return 0; } 输出结果: Line Rect
时间: 2024-02-01 18:03:36 浏览: 52
这段代码存在问题,因为在 main 函数中定义了两个名为 p 的指针变量,这样会导致编译错误。
另外,类 Rect 中的 show 函数名应该改为 Show,以保持与基类 Shape 中的虚函数名一致。修改后的代码如下:
```
#include<iostream>
using namespace std;
class Shape{
public:
virtual void Show() = 0;
};
class Line: public Shape{
public:
void Show(){ cout << "Line" << endl; }
};
class Rect: public Shape{
public:
void Show(){ cout << "Rect" << endl; }
};
int main(){
Shape *p = new Line();
p->Show();
delete p;
p = new Rect();
p->Show();
delete p;
return 0;
}
```
输出结果为:
```
Line
Rect
```
其中,Shape 类中的 Show 函数是纯虚函数,因此 Line 和 Rect 类都需要实现该函数并重写基类中的该函数。在 main 函数中,先创建一个指向 Line 类对象的 Shape 指针 p,调用 p 的 Show 函数输出 "Line",然后释放内存;再创建一个指向 Rect 类对象的 Shape 指针 p,调用 p 的 Show 函数输出 "Rect",最后再释放内存。
相关推荐
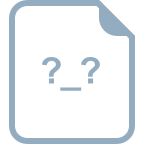










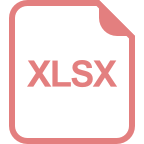
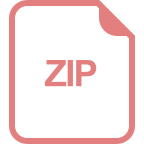
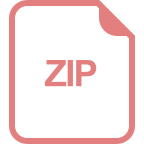