根据大数定理预测双色球号码的c#代码
时间: 2023-07-28 08:10:28 浏览: 66
大数定理一般用于统计学上的分析,不适用于预测双色球号码。预测双色球号码需要结合历史开奖数据和一定的算法来进行分析和预测。以下是一个简单的基于历史数据的预测算法的C#代码,仅供参考:
```
using System;
using System.Collections.Generic;
using System.Linq;
namespace DoubleColorBallPredictor
{
class Program
{
static void Main(string[] args)
{
// 历史开奖数据
int[][] history = new int[][] {
new int[] { 1, 5, 8, 17, 21, 28, 10 },
new int[] { 3, 9, 14, 18, 24, 29, 4 },
new int[] { 7, 10, 13, 15, 17, 18, 10 },
// ...
};
// 获取所有红球号码
List<int> redBalls = new List<int>();
foreach (var item in history)
{
redBalls.AddRange(item.Take(6));
}
// 获取所有蓝球号码
List<int> blueBalls = new List<int>();
foreach (var item in history)
{
blueBalls.Add(item[6]);
}
// 统计红球出现次数
Dictionary<int, int> redCounts = new Dictionary<int, int>();
foreach (var item in redBalls)
{
if (redCounts.ContainsKey(item))
{
redCounts[item]++;
}
else
{
redCounts[item] = 1;
}
}
// 统计蓝球出现次数
Dictionary<int, int> blueCounts = new Dictionary<int, int>();
foreach (var item in blueBalls)
{
if (blueCounts.ContainsKey(item))
{
blueCounts[item]++;
}
else
{
blueCounts[item] = 1;
}
}
// 根据出现次数排序
var redSorted = redCounts.OrderByDescending(x => x.Value).Select(x => x.Key);
var blueSorted = blueCounts.OrderByDescending(x => x.Value).Select(x => x.Key);
Console.WriteLine("红球预测:{0} {1} {2} {3} {4} {5}", redSorted.Take(6).ToArray());
Console.WriteLine("蓝球预测:{0}", blueSorted.First());
}
}
}
```
该代码根据历史开奖数据统计每个号码出现的次数,然后按照出现次数排序,选择出现次数较多的号码作为预测结果。这种算法的准确度不高,仅供娱乐参考。
相关推荐
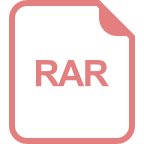














