用C++写一个带有增加快递 删除快递 管理员 的快递管理系统
时间: 2023-10-06 22:09:12 浏览: 45
好的,我可以帮你设计一个简单的快递管理系统。首先,我们需要确定系统的功能和数据结构。
系统功能:
1. 增加快递:输入快递单号和收件人信息,将其保存到系统中
2. 删除快递:根据快递单号删除快递信息
3. 管理员:需要管理员密码才能进行删除操作
数据结构:
1. 快递信息:包括快递单号和收件人信息
2. 快递列表:用链表或数组保存所有快递信息
3. 管理员密码:保存在程序中
根据上述功能和数据结构,我们可以设计以下的 C++ 代码:
```cpp
#include <iostream>
#include <string>
#include <cstring>
using namespace std;
// 定义快递信息结构体
struct ExpressInfo {
string id; // 快递单号
string name; // 收件人姓名
string phone; // 收件人电话
};
// 定义快递节点结构体
struct ExpressNode {
ExpressInfo info; // 快递信息
ExpressNode* next; // 下一个节点指针
};
// 定义快递管理系统类
class ExpressManager {
private:
ExpressNode* head; // 快递列表头指针
string password; // 管理员密码
public:
// 构造函数
ExpressManager() {
head = NULL;
password = "123456"; // 默认密码
}
// 增加快递
void addExpress() {
// 输入快递信息
ExpressInfo info;
cout << "请输入快递单号:";
cin >> info.id;
cout << "请输入收件人姓名:";
cin >> info.name;
cout << "请输入收件人电话:";
cin >> info.phone;
// 创建快递节点
ExpressNode* node = new ExpressNode;
node->info = info;
node->next = NULL;
// 将节点加入快递列表
if (head == NULL) {
head = node;
} else {
ExpressNode* p = head;
while (p->next != NULL) {
p = p->next;
}
p->next = node;
}
cout << "快递添加成功!" << endl;
}
// 删除快递
void deleteExpress() {
string id;
cout << "请输入要删除的快递单号:";
cin >> id;
// 查找快递节点
ExpressNode* p = head;
ExpressNode* pre = NULL;
while (p != NULL) {
if (p->info.id == id) {
break;
}
pre = p;
p = p->next;
}
if (p == NULL) {
cout << "没有找到该快递!" << endl;
} else {
// 判断是否需要管理员权限
string inputPassword;
if (pre == NULL) { // 删除头节点需要管理员权限
cout << "请输入管理员密码:";
cin >> inputPassword;
if (inputPassword != password) {
cout << "管理员密码错误,无法删除该快递!" << endl;
return;
}
head = p->next;
} else { // 删除非头节点不需要管理员权限
pre->next = p->next;
}
delete p;
cout << "快递删除成功!" << endl;
}
}
};
int main() {
ExpressManager manager;
while (true) {
cout << "请选择操作:1.增加快递 2.删除快递 3.退出" << endl;
int choice;
cin >> choice;
switch (choice) {
case 1:
manager.addExpress();
break;
case 2:
manager.deleteExpress();
break;
case 3:
return 0;
default:
cout << "输入有误,请重新输入!" << endl;
}
}
return 0;
}
```
上述代码实现了增加快递和删除快递的功能,管理员密码默认为 "123456"。你可以根据需要进行修改和扩展。
相关推荐
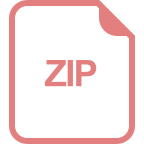














