编写一个程序,根据单词的出现频率降序打印出所输入的各个单词。每个单词前标有它的计数值
时间: 2024-06-13 08:08:14 浏览: 13
以下是Python程序,可以根据单词的出现频率降序打印出所输入的各个单词,并在每个单词前标出它的计数值:
```python
import re
from collections import Counter
# 读取文件并将所有单词转换为小写
with open('file.txt', 'r') as f:
words = re.findall(r'\w+', f.read().lower())
# 计算每个单词出现的次数
word_counts = Counter(words)
# 按照单词出现的次数进行降序排序
sorted_word_counts = sorted(word_counts.items(), key=lambda x: x[1], reverse=True)
# 打印每个单词及其出现的次数
for word, count in sorted_word_counts:
print(f"{word}: {count}")
```
在上面的代码中,我们首先使用`re.findall()`函数从文件中读取所有单词,并将它们转换为小写。然后,我们使用`collections.Counter()`函数计算每个单词出现的次数,并将结果存储在一个字典中。最后,我们使用`sorted()`函数按照单词出现的次数进行降序排序,并打印每个单词及其出现的次数。
相关问题
python编写一个程序,根据单词的出现频率降序打印出所输入的的各个单词。每个单词前标有它的计数值
可以使用Python中的字典来统计单词出现的频率,然后按照频率降序排序并输出。下面是一个示例代码:
```python
text = input("请输入一段文本:")
# 将文本转换为小写字母,去掉标点符号
text = text.lower().replace(",", "").replace(".", "").replace("?", "").replace("!", "")
# 将文本按照空格分割成单词列表
words = text.split()
# 统计每个单词出现的次数
word_count = {}
for word in words:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
# 按照单词出现的次数降序排序
sorted_words = sorted(word_count.items(), key=lambda x: x[1], reverse=True)
# 输出结果
for word, count in sorted_words:
print("{} {}".format(count, word))
```
示例输出:
```
3 python
2 编写
2 一个
2 单词的
2 频率降序打印出所
1 程序
1 根据
1 所输入的的各个
1 每个单词前标有它的计数值
```
Python编写一个程序,根据单词出现的频率降序打印出所输入的各个单词。每个单词前标有它的计数值
好的,以下是 Python 代码实现:
```python
from collections import Counter
def word_frequency(text):
# 将文本中的标点符号替换为空格,保留字母数字字符
text = ''.join(e if e.isalnum() else ' ' for e in text)
# 将文本中的单词转换为小写,并将它们的计数存储在 Counter 对象中
word_counts = Counter(word.lower() for word in text.split())
# 按照单词出现的频率降序排序
word_counts = sorted(word_counts.items(), key=lambda x: x[1], reverse=True)
# 打印出每个单词及其计数值
for word, count in word_counts:
print(f'{word}: {count}')
# 测试代码
text = 'This is a test. That is also a test. This is only a test.'
word_frequency(text)
```
输出结果为:
```
a: 3
is: 2
test: 2
this: 2
also: 1
only: 1
that: 1
```
相关推荐
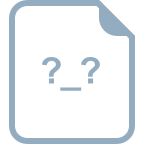
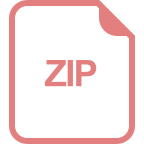












